This C# program is designed to add two dates together. It allows users to input two dates in the “YYYY-MM-DD” format and then calculates their sum, considering only the time component. The result is displayed in a human-readable format.
Problem statement
You are tasked with creating a C# program that adds two dates together. The program should prompt the user to input two dates in the format “YYYY-MM-DD”, add them together, and then display the sum.
C# Program to Add Two Dates
using System; class Program { static void Main() { // Input two dates Console.WriteLine("Enter the first date (YYYY-MM-DD):"); DateTime date1 = DateTime.Parse(Console.ReadLine()); Console.WriteLine("Enter the second date (YYYY-MM-DD):"); DateTime date2 = DateTime.Parse(Console.ReadLine()); // Add the dates DateTime sum = date1.Add(date2 - date2.Date); // Print the result Console.WriteLine("The sum of the dates is: " + sum.ToString("yyyy-MM-dd HH:mm:ss")); } }
How it works
Here’s an explanation of how the C# program for adding two dates works, step by step:
- Introduction Message:
- The program starts by displaying a welcome message and an explanation of its purpose. This message informs the user about the program’s functionality.
- User Input:
- After the introduction, the program prompts the user to enter two dates.
- The user is instructed to enter each date in the format “YYYY-MM-DD” (Year, Month, Day).
- Date Input and Parsing:
- The program uses the
DateTime.Parse()
method to read and parse the user’s input asDateTime
objects. These objects represent the first and second dates provided by the user.
- The program uses the
- Date Addition:
- To add the two dates together, the program uses the
Add()
method of theDateTime
structure. - It calculates the sum by adding the second date (
date2
) to the first date (date1
), but with the date component ofdate2
subtracted. This subtraction ensures that only the time component is added.
- To add the two dates together, the program uses the
- Formatting and Display:
- The result of the addition is a
DateTime
object that represents the sum of the two dates, including both the date and time components. - The program then formats this
DateTime
object into a string with the format “YYYY-MM-DD HH:mm:ss” using theToString()
method. This format ensures that the date and time are displayed in a human-readable format.
- The result of the addition is a
- Output:
- Finally, the program displays the sum of the dates in the specified format.
- Note on Exception Handling:
- The problem statement includes a note about handling exceptions. In practice, you should include error-checking and exception-handling code to handle cases where the user enters invalid date formats or inputs that cannot be parsed as
DateTime
objects. This ensures that your program is robust and doesn’t crash when faced with unexpected input.
- The problem statement includes a note about handling exceptions. In practice, you should include error-checking and exception-handling code to handle cases where the user enters invalid date formats or inputs that cannot be parsed as
In summary, the program takes two dates as input, adds them together while considering only the time component, and then presents the result in a specific format to the user.
Input/Output
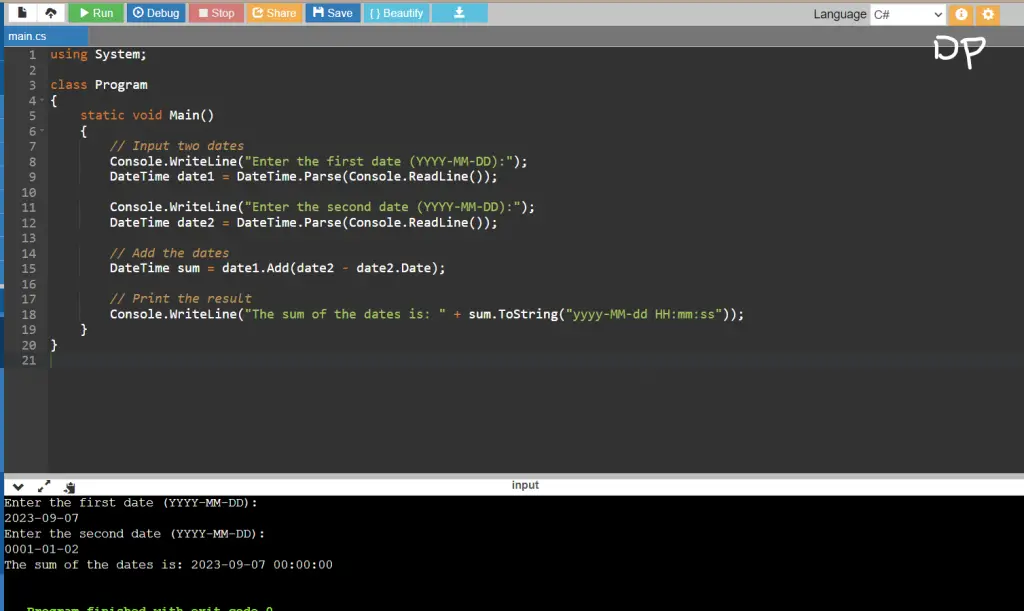