This C# program checks whether a given number is an amicable number or not. An amicable number is a pair of numbers such that the sum of the proper divisors of each number is equal to the other number in the pair. The program calculates the sum of proper divisors for the given number and its sum of divisors, and then compares them to determine if the number is amicable or not. It prompts the user to enter a number and provides the result of the amicable number check.
Problem Statement
Write a C# program to check whether a given pair of numbers forms an amicable pair. An amicable pair consists of two positive integers, where the sum of the proper divisors of the first number equals the second number, and vice versa. For example, (220, 284) is an amicable pair because the sum of divisors of 220 is 284, and the sum of divisors of 284 is 220.
C# Program to Check Whether the Given Number is a Amicable Number or Not
using System; class Program { static void Main() { Console.Write("Enter the first number: "); int num1 = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter the second number: "); int num2 = Convert.ToInt32(Console.ReadLine()); if (AreAmicableNumbers(num1, num2)) { Console.WriteLine($"({num1}, {num2}) is an amicable pair."); } else { Console.WriteLine($"({num1}, {num2}) is not an amicable pair."); } } static bool AreAmicableNumbers(int num1, int num2) { if (num1 <= 0 || num2 <= 0) { return false; } int sumDivisors1 = CalculateSumOfDivisors(num1); int sumDivisors2 = CalculateSumOfDivisors(num2); return sumDivisors1 == num2 && sumDivisors2 == num1; } static int CalculateSumOfDivisors(int num) { int sum = 0; for (int divisor = 1; divisor < num; divisor++) { if (num % divisor == 0) { sum += divisor; } } return sum; } }
Input / Output
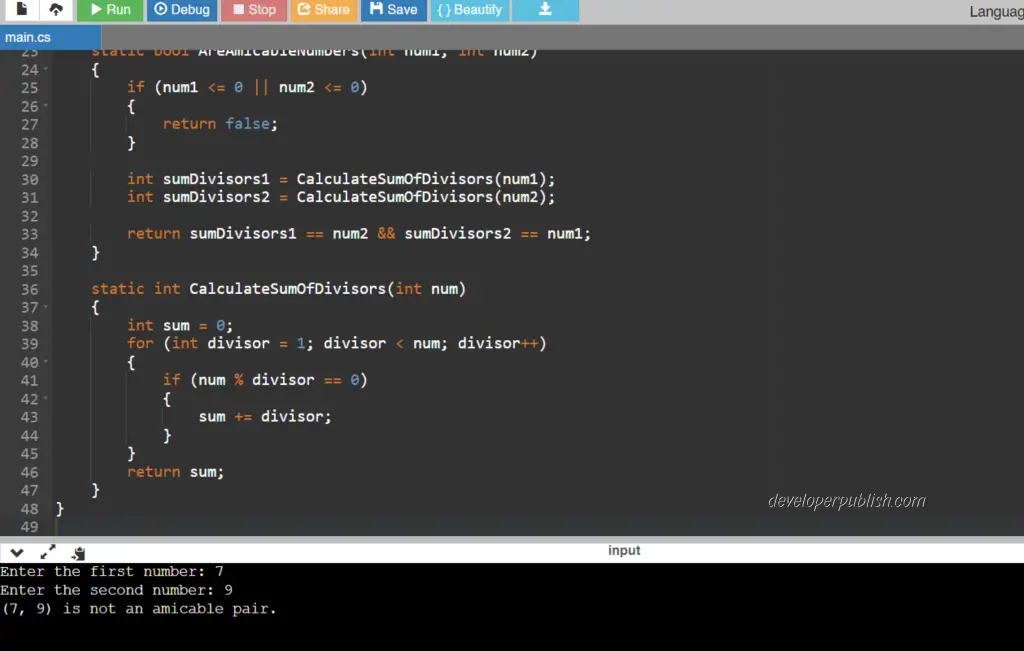