This C# program calculates the sum of digits of a given number using a recursive function.
Problem statement
You are given a positive integer n
. Your task is to write a recursive function to calculate the sum of its digits.
C# Program to Find Sum of Digits of a Number using Recursion
using System; class Program { // Function to calculate the sum of digits using recursion static int SumOfDigits(int number) { // Base case: If the number is a single digit, return the number itself if (number < 10) { return number; } else { // Get the last digit int lastDigit = number % 10; // Recursively calculate the sum of digits for the remaining part of the number int remainingSum = SumOfDigits(number / 10); // Add the last digit to the sum of the remaining digits int totalSum = lastDigit + remainingSum; return totalSum; } } static void Main(string[] args) { Console.Write("Enter a number: "); int number = int.Parse(Console.ReadLine()); // Calculate the sum of digits using the SumOfDigits function int sum = SumOfDigits(number); Console.WriteLine($"The sum of digits of {number} is {sum}"); } }
How it works
Sure, let’s break down how the recursive program to calculate the sum of digits works step by step:
- Input: The program takes an integer
n
as input. - Recursive Function: The program defines a recursive function called
SumOfDigits
, which is responsible for calculating the sum of the digits of the input number.
- Base Case: Inside the
SumOfDigits
function, there is a base case check. If thenumber
is less than 10, it means thatnumber
is a single digit (0-9). In this case, we return the number itself because the sum of a single digit is the digit itself. - Recursive Case: If the
number
is not a single digit (greater than or equal to 10), we proceed to calculate the sum of its digits recursively:- We calculate the
lastDigit
by taking the remainder ofnumber
when divided by 10. This gives us the last digit of the number. - We calculate the
remainingSum
by calling theSumOfDigits
function recursively with the integer division ofnumber
by 10. This effectively removes the last digit fromnumber
and focuses on the remaining part of the number. - We calculate the
totalSum
by addinglastDigit
to theremainingSum
.
- We calculate the
- Return: The
totalSum
is returned as the result of theSumOfDigits
function. - Main Function: In the
Main
method of the program, the user is prompted to enter an integern
. Then, theSumOfDigits
function is called withn
as an argument. - Output: Finally, the program displays the sum of digits, which is the result returned by the
SumOfDigits
function.
The recursion works by breaking down the input number into its individual digits, calculating their sum, and then applying the same process to the remaining part of the number until the base case is reached (when the number becomes a single digit). At that point, the base case handles the termination of the recursion by returning the single digit itself.
Input/Output
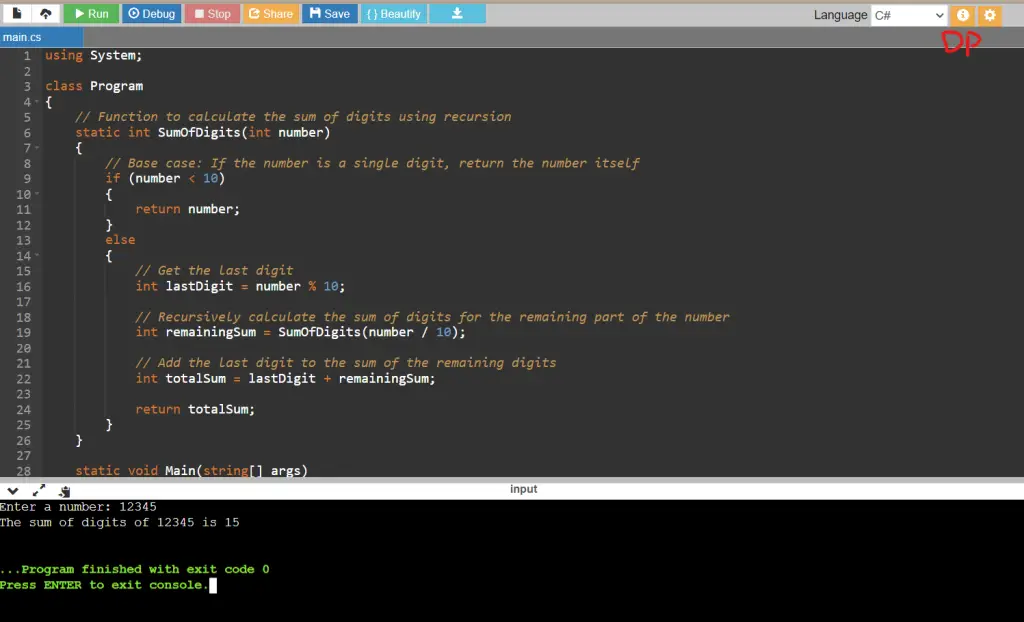