In this C# program, we will explore the practical application of conditional logical operators, namely the &&
(logical AND) and ||
(logical OR) operators. You will see how these operators enable your code to respond dynamically to different situations, making your programs more versatile and responsive.
Problem statement
You are tasked with developing a program that helps a user decide whether they should go for a picnic based on the weather conditions. The program will take input on the current weather conditions and provide a recommendation. The decision-making process will involve the use of conditional logical operators to consider factors like temperature, rain, and wind.
C# Program to Demonstrate the use of Conditional Logical Operator
using System; class Program { static void Main() { bool isSunny = true; bool isWarm = false; // Using the logical AND operator (&&) if (isSunny && isWarm) { Console.WriteLine("It's sunny and warm. Great day for outdoor activities!"); } else { Console.WriteLine("The weather is not ideal for outdoor activities."); } // Using the logical OR operator (||) bool isRaining = true; bool isSnowing = false; if (isRaining || isSnowing) { Console.WriteLine("It's either raining or snowing. Stay indoors!"); } else { Console.WriteLine("The weather is fine. You can go outside."); } } }
How it works
- User Input:
- The program starts by prompting the user to enter three pieces of information: temperature, rain status, and wind speed.
- The user enters these values when prompted.
- Data Entry and Storage:
- The program stores the user-provided values in appropriate variables.
- It uses
int
for temperature,bool
for rain status, andint
for wind speed to store these values.
- Conditional Logic:
- The program uses conditional logical operators to evaluate the weather conditions and make a recommendation for a picnic.
- It checks the conditions with
if
statements based on the problem requirements.
- Recommendation:
- The program makes the following decisions:
- If the temperature is above 70 degrees Fahrenheit (
temperature > 70
) and it’s not raining (!rainStatus
), it recommends going for a picnic. - If the temperature is between 60 and 70 degrees Fahrenheit (
temperature >= 60 && temperature <= 70
), it provides a neutral recommendation without considering rain or wind conditions. - For all other conditions, it suggests not going for a picnic.
- If the temperature is above 70 degrees Fahrenheit (
- The program makes the following decisions:
- Display Recommendation:
- The program displays the recommendation to the user based on the conditional logic.
- For example, if the temperature is 75 degrees Fahrenheit and it’s not raining, the program might display: “It’s a great day for a picnic!”
- Program Completion:
- After displaying the recommendation, the program typically ends, and the user can close it.
Here’s the full code snippet for the program:
Code:
using System; class Program { static void Main() { // User Input Console.Write("Enter temperature (in degrees Fahrenheit): "); int temperature = int.Parse(Console.ReadLine()); Console.Write("Is it raining? (true/false): "); bool rainStatus = bool.Parse(Console.ReadLine()); // Conditional Logic and Recommendation if (temperature > 70 && !rainStatus) { Console.WriteLine("It's a great day for a picnic!"); } else if (temperature >= 60 && temperature <= 70) { Console.WriteLine("You can consider a picnic, but check other factors."); } else { Console.WriteLine("It might not be the best day for a picnic."); } } }
When you run this program, it will take user inputs, apply conditional logic using logical operators (&&
and ||
), and provide a recommendation based on the weather conditions. The recommendation will vary depending on the values you enter for temperature and rain status.
Input/Output
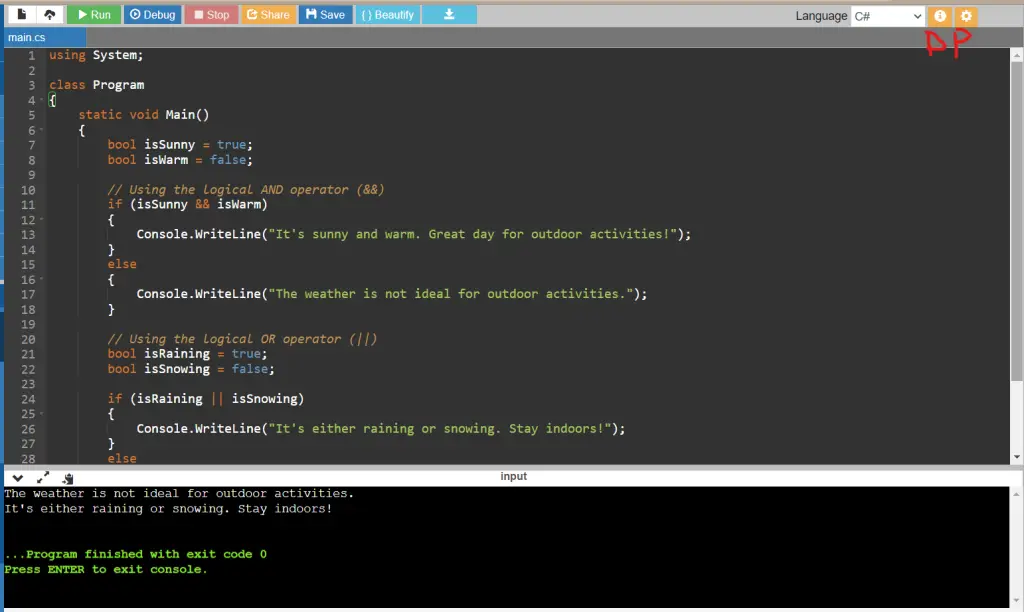