In this c# program, we will explore a selection of data types and display their minimum and maximum values, offering valuable insights into their characteristics.
Problem statement
You are tasked with creating a C# program that determines and displays the minimum and maximum values for a selection of fundamental data types. Understanding the range of values that each data type can hold is crucial for efficient programming and preventing data overflow or underflow errors.
C# Program to Find the Minimum Range of Data Types
using System; class Program { static void Main() { Console.WriteLine("Minimum Range of Data Types:"); Console.WriteLine($"sbyte: {sbyte.MinValue} to {sbyte.MaxValue}"); Console.WriteLine($"byte: {byte.MinValue} to {byte.MaxValue}"); Console.WriteLine($"short: {short.MinValue} to {short.MaxValue}"); Console.WriteLine($"ushort: {ushort.MinValue} to {ushort.MaxValue}"); Console.WriteLine($"int: {int.MinValue} to {int.MaxValue}"); Console.WriteLine($"uint: {uint.MinValue} to {uint.MaxValue}"); Console.WriteLine($"long: {long.MinValue} to {long.MaxValue}"); Console.WriteLine($"ulong: {ulong.MinValue} to {ulong.MaxValue}"); Console.WriteLine($"float: {float.MinValue} to {float.MaxValue}"); Console.WriteLine($"double: {double.MinValue} to {double.MaxValue}"); Console.WriteLine($"decimal: {decimal.MinValue} to {decimal.MaxValue}"); } }
How it works
To understand how the C# program for finding the minimum range of data types works, let’s break down its key components and logic step by step:
- Importing the Necessary Namespace:The program starts by importing the
System
namespace, which provides access to essential C# libraries and classes required for various functionalities.
Code: using System;
Defining the Main
Method:
Every C# program starts execution from the Main
method. In this case, the Main
method serves as the entry point for the program.
Code:
static void Main()
{
// Program logic goes here
}
Displaying an Introduction:
Before diving into the core functionality, the program displays an introduction that explains the purpose and significance of the program. It sets the context for what the program aims to achieve.
Displaying Data Type Ranges:
The program proceeds to display the minimum and maximum values for a selection of fundamental data types. It does so by utilizing the MinValue
and MaxValue
properties of each data type.
For example, for the sbyte
data type:
Code: Console.WriteLine($”sbyte: {sbyte.MinValue} to {sbyte.MaxValue}”);
- This line of code prints the minimum and maximum values for the
sbyte
data type. - Repeating for Other Data Types:The program repeats the above process for each of the specified data types, such as
byte
,short
,ushort
, and so on. - Displaying Output:The program prints the data type ranges in a clear and organized manner, often using string interpolation (
$
) to format the output neatly. - Conclusion:After displaying the ranges for all data types, the program effectively concludes.
- Running the Program:When you run this program, it will execute the
Main
method, and you will see the output on the console. The output will consist of the minimum and maximum values for the specified data types.
By following these steps, the program provides a quick reference for the minimum ranges of various data types in C#. It demonstrates how to use these data types and their associated properties to obtain crucial information for programming tasks, ensuring data integrity and preventing errors related to data overflow or underflow.
Input/Output
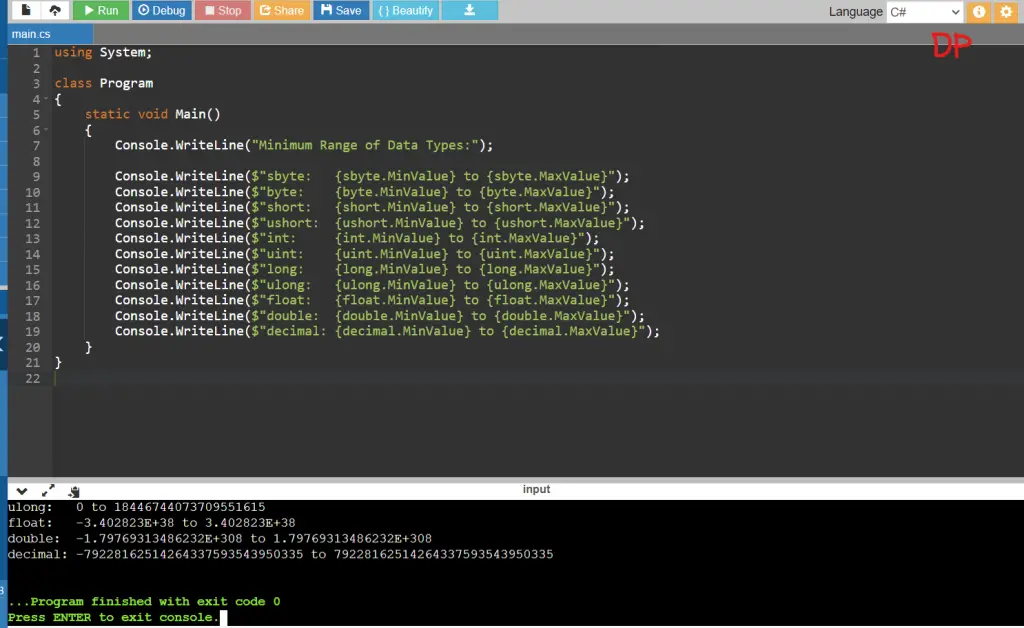