Creating a C# program to implement a phonebook is a common beginner-level project that can help you learn the basics of C# programming. In this program, you’ll typically define a data structure to store contacts, and then you’ll provide functionalities to add, view, search, and delete contacts.
Problem statement
You are tasked with creating a simple console-based phonebook application in C#. The program should allow users to manage their contacts by providing functionalities to add, view, search, and delete contacts.
C# Program to Implement PhoneBook
using System; using System.Collections.Generic; class Contact { public string Name { get; set; } public string PhoneNumber { get; set; } } class PhoneBook { private List<Contact> contacts = new List<Contact>(); public void AddContact(Contact contact) { contacts.Add(contact); } public void ViewAllContacts() { Console.WriteLine("All Contacts:"); foreach (var contact in contacts) { Console.WriteLine($"Name: {contact.Name}, Phone Number: {contact.PhoneNumber}"); } Console.WriteLine(); } public Contact SearchContact(string name) { return contacts.Find(contact => contact.Name.Equals(name, StringComparison.OrdinalIgnoreCase)); } public void DeleteContact(string name) { Contact contactToRemove = SearchContact(name); if (contactToRemove != null) { contacts.Remove(contactToRemove); Console.WriteLine($"{name} has been deleted from the phonebook."); } else { Console.WriteLine($"{name} was not found in the phonebook."); } } } class Program { static void Main() { PhoneBook phoneBook = new PhoneBook(); while (true) { Console.WriteLine("1. Add Contact"); Console.WriteLine("2. View All Contacts"); Console.WriteLine("3. Search Contact"); Console.WriteLine("4. Delete Contact"); Console.WriteLine("5. Exit"); Console.Write("Enter your choice: "); int choice = int.Parse(Console.ReadLine()); switch (choice) { case 1: Console.Write("Enter Name: "); string name = Console.ReadLine(); Console.Write("Enter Phone Number: "); string phoneNumber = Console.ReadLine(); Contact newContact = new Contact { Name = name, PhoneNumber = phoneNumber }; phoneBook.AddContact(newContact); Console.WriteLine($"{name} has been added to the phonebook.\n"); break; case 2: phoneBook.ViewAllContacts(); break; case 3: Console.Write("Enter Name to search: "); string searchName = Console.ReadLine(); Contact foundContact = phoneBook.SearchContact(searchName); if (foundContact != null) { Console.WriteLine($"Name: {foundContact.Name}, Phone Number: {foundContact.PhoneNumber}\n"); } else { Console.WriteLine($"{searchName} was not found in the phonebook.\n"); } break; case 4: Console.Write("Enter Name to delete: "); string deleteName = Console.ReadLine(); phoneBook.DeleteContact(deleteName); break; case 5: Environment.Exit(0); break; default: Console.WriteLine("Invalid choice. Please try again.\n"); break; } } } }
How it works
Here’s a step-by-step explanation of how the phonebook program in C# works based on the provided problem statement:
- Contact Class:
- We start by defining a
Contact
class that represents a single contact with two properties:Name
(to store the contact’s name as a string) andPhoneNumber
(to store the contact’s phone number as a string).
- We start by defining a
Code:
class Contact
{
public string Name { get; set; }
public string PhoneNumber { get; set; }
}
PhoneBook Class:
- Next, we create a
PhoneBook
class that is responsible for managing contacts. It has several methods:AddContact(Contact contact)
: This method takes aContact
object as an argument and adds it to a list of contacts within the phonebook.ViewAllContacts()
: This method displays all contacts stored in the phonebook by iterating through the list and printing their names and phone numbers.SearchContact(string name)
: Given a name, this method searches for a contact with that name in the phonebook and returns it. If the contact is not found, it returnsnull
.DeleteContact(string name)
: This method deletes a contact from the phonebook based on the given name. If the contact is found and deleted, it prints a message. If not found, it prints a message indicating that the contact was not found.
Code:
class PhoneBook
{
private List contacts = new List();
// Methods to add, view, search, and delete contacts // ...
}
- Main Program:
- In the
Main
method of the program, we create an instance of thePhoneBook
class to manage our contacts. - We enter a loop that continuously displays a menu of options to the user:
1. Add Contact
: Allows the user to add a new contact by entering the name and phone number.2. View Contacts
: Displays all the contacts currently stored in the phonebook.3. Search Contact
: Allows the user to search for a contact by entering a name. It either displays the contact’s details or indicates that the contact was not found.4. Delete Contact
: Allows the user to delete a contact by entering a name. It either deletes the contact or indicates that the contact was not found.5. Exit
: Allows the user to exit the program.
- Depending on the user’s choice, the program calls the corresponding method in the
PhoneBook
class to perform the desired action.
- In the
- Data Validation and Error Handling:
- Throughout the program, we implement data validation to ensure that the user’s input is correct. For example, when adding a contact, we may validate that the phone number is in the correct format.
- We also handle potential issues, such as attempting to add a duplicate contact or trying to delete a contact that does not exist. In such cases, appropriate error messages are displayed to the user.
- Exit Mechanism:
- We provide the user with an option (choice 5) to exit the program gracefully by using
Environment.Exit(0)
.
- We provide the user with an option (choice 5) to exit the program gracefully by using
- Example Output:
- The program demonstrates its functionality by displaying a menu to the user, allowing them to interact with the phonebook, and providing feedback based on their choices.
By following these steps, the program allows users to manage their contacts effectively by adding, viewing, searching, and deleting contacts within the phonebook.
Input/Output
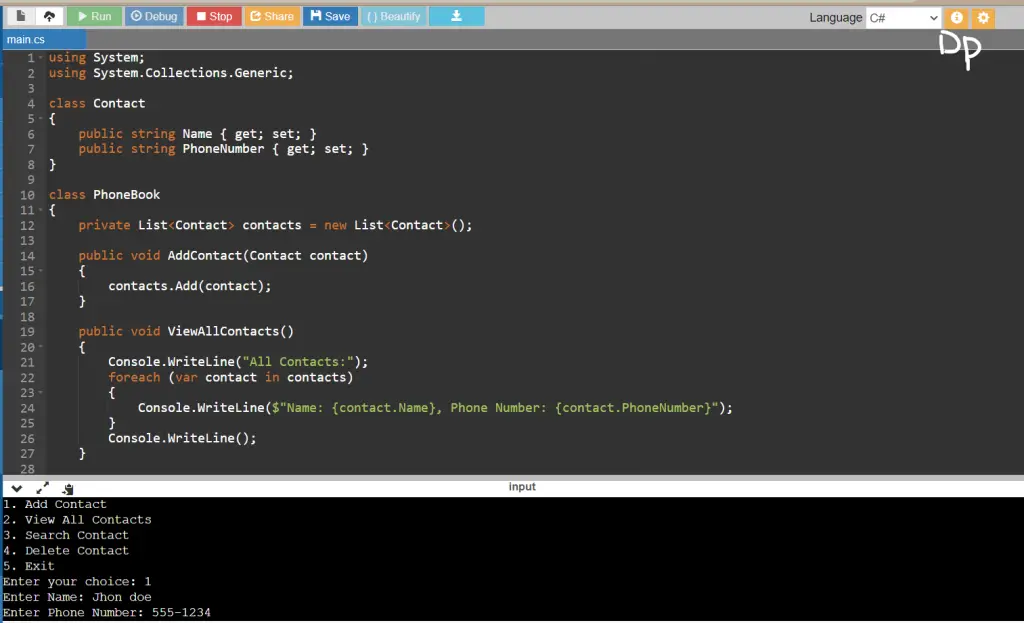