In this C program, we will be adding two complex numbers. A complex number consists of a real part and an imaginary part. We will take input from the user for the real and imaginary parts of both complex numbers and then add them together.
Problem Statements
Write a C program to add two complex numbers.
C Program to Add Two Complex Numbers
#include<stdio.h> typedef struct { float real; float imaginary; } Complex; Complex addComplexNumbers(Complex num1, Complex num2); int main() { Complex num1, num2, sum; printf("Enter real and imaginary parts of first complex number:\n"); printf("Real Part: "); scanf("%f", &num1.real); printf("Imaginary Part: "); scanf("%f", &num1.imaginary); printf("\nEnter real and imaginary parts of second complex number:\n"); printf("Real Part: "); scanf("%f", &num2.real); printf("Imaginary Part: "); scanf("%f", &num2.imaginary); sum = addComplexNumbers(num1, num2); printf("\nSum = %.2f + %.2fi", sum.real, sum.imaginary); return 0; } Complex addComplexNumbers(Complex num1, Complex num2) { Complex sum; sum.real = num1.real + num2.real; sum.imaginary = num1.imaginary + num2.imaginary; return sum; }
How it works
- We start by including the necessary header file
stdio.h
, which allows us to use functions likeprintf
andscanf
. - We define a structure called
Complex
that represents a complex number. It contains two float variables:real
for the real part andimaginary
for the imaginary part. - We declare a function
addComplexNumbers
that takes two complex numbers as parameters and returns the sum of the two numbers. - In the
main
function, we declare three variables:num1
andnum2
to store the two complex numbers entered by the user, andsum
to store the sum of the complex numbers. - We prompt the user to enter the real and imaginary parts of the first complex number, and we read the values using
scanf
. - Similarly, we prompt the user to enter the real and imaginary parts of the second complex number, and we read the values using
scanf
. - We call the
addComplexNumbers
function, passingnum1
andnum2
as arguments, and assign the returned value to thesum
variable. - Finally, we display the sum of the two complex numbers using
printf
, formatting it as%.2f + %.2fi
to display the real and imaginary parts with two decimal places.
Input / Output
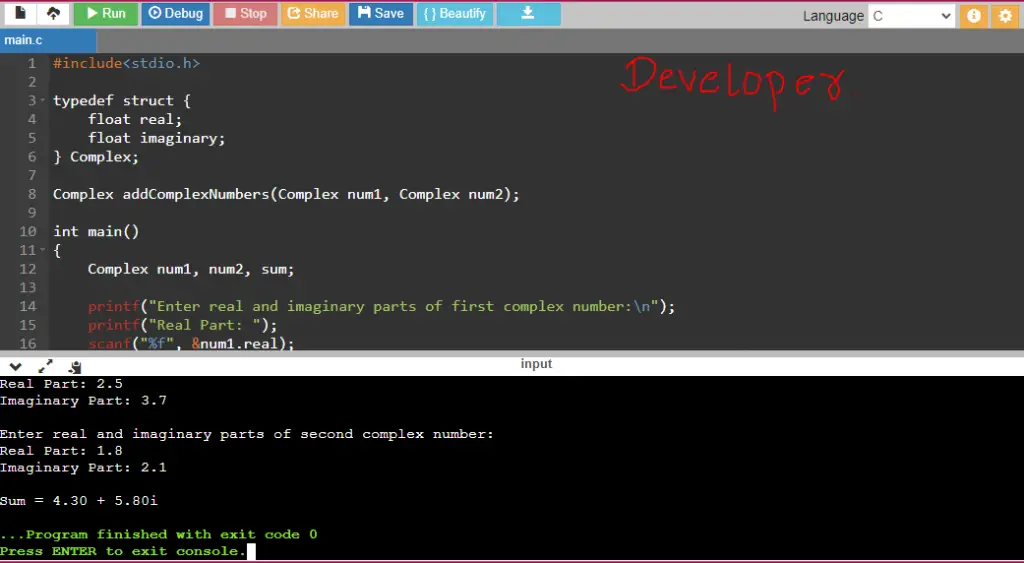