This Python program counts the number of uppercase and lowercase letters in a string.
Problem statement
You are tasked with writing a Python program that takes a string as input and counts the number of uppercase and lowercase letters in the string. Your program should then display the counts of both uppercase and lowercase letters separately.
Python Program to Count Number of Uppercase and Lowercase Letters in a String
# Function to count uppercase and lowercase letters in a string def count_letters(string): # Initialize counters for uppercase and lowercase letters uppercase_count = 0 lowercase_count = 0 # Iterate through each character in the string for char in string: # Check if the character is an uppercase letter if char.isupper(): uppercase_count += 1 # Check if the character is a lowercase letter elif char.islower(): lowercase_count += 1 return uppercase_count, lowercase_count # Input string input_string = input("Enter a string: ") # Call the count_letters function uppercase, lowercase = count_letters(input_string) # Display the results print("Uppercase letters:", uppercase) print("Lowercase letters:", lowercase)
How it works
The Python program provided in response to the problem statement works as follows:
- Function Definition (
count_upper_lower
): First, a function namedcount_upper_lower
is defined. This function takes one parameter, which is the input string for which we want to count uppercase and lowercase letters. - Counter Initialization: Inside the
count_upper_lower
function, two counters,upper_count
andlower_count
, are initialized to zero. These counters will be used to keep track of the number of uppercase and lowercase letters in the string. - Character Iteration: The program then iterates through each character in the input string using a
for
loop. It processes one character at a time. - Uppercase and Lowercase Check: For each character in the string, the program checks whether it’s an uppercase letter using the
isupper()
method and whether it’s a lowercase letter using theislower()
method.- If the character is an uppercase letter, the
upper_count
is incremented by 1. - If the character is a lowercase letter, the
lower_count
is incremented by 1.
- If the character is an uppercase letter, the
- Returning Counts: After processing all characters in the string, the function returns a tuple containing the counts of uppercase and lowercase letters. This tuple contains two values:
upper_count
andlower_count
. - Input from User: In the main part of the program, it asks the user to input a string using the
input()
function and stores it in theinput_string
variable. - Function Call: The
count_upper_lower
function is called withinput_string
as an argument to count the uppercase and lowercase letters in the input string. - Displaying Results: Finally, the program displays the counts of uppercase and lowercase letters to the user using the
print()
function.
The program follows this logic to count the uppercase and lowercase letters in the input string, and it displays the results in a user-friendly format.
Input/Output
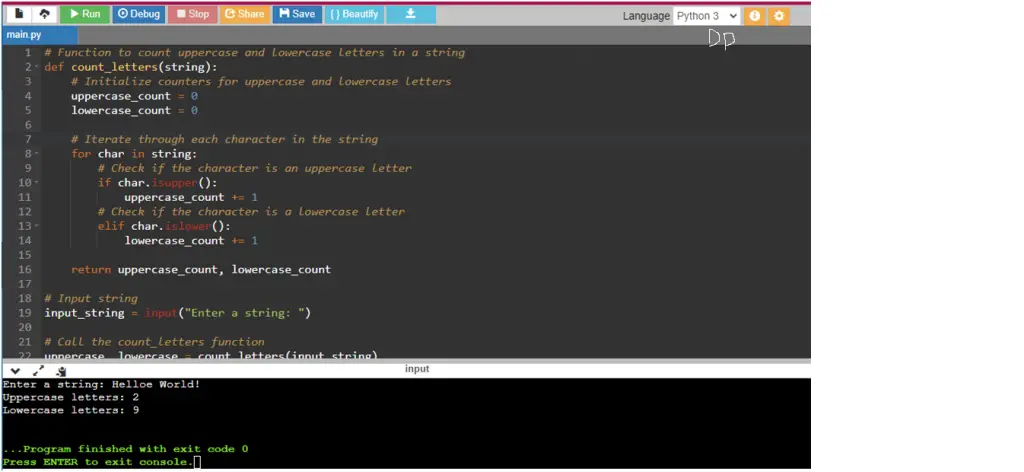