This python program compares two strings and displays the letters that are present in the first string but not in the second.
Problem Statement
Given two strings, the task is to find and display the letters that are present in the first string but not in the second string using python programming language.
Python Program that Displays which Letters are in First String but not in Second
def find_unique_letters( first_string, second_string): unique_letters = "" for char in first_string: if char not in second_string and char not in unique_letters: unique_letters += char return unique_letters second_string = input("Enter the second string: ") first_string = input("Enter the first string: ") result = find_unique_letters(first_string, second_string) print("Letters in the first string but not in the second:", result)
How It Works
- The program defines a function called “find_unique_letters” that takes two input strings: first_string and second_string.
- It initializes an empty string called “unique_letters” to store the letters that are present in the first_string but not in the second_string.
- The program then iterates through each character in the first_string.
- If the character is not in the second_string and has not already been added to the unique_letters, it is added.
- Finally, the unique_letters string is returned, containing the letters that meet the specified criteria.
Input / Output
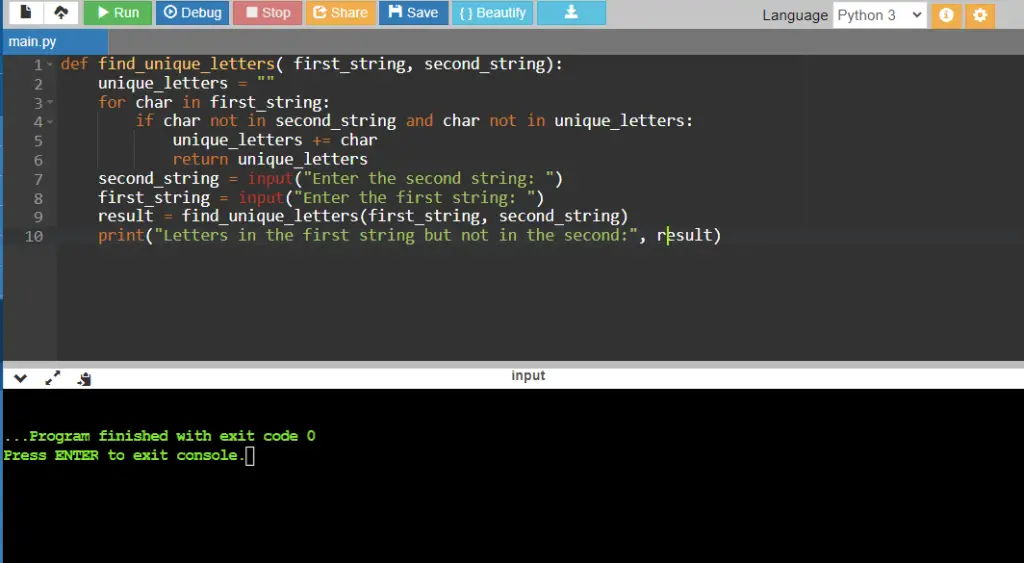