In this program, we will write a Python program to find the number that occurs an odd number of times in a given list of integers. We will use a simple approach and bitwise XOR operation to solve this problem efficiently.
Problem Statement
Given a list of integers, where all numbers occur even times except for one number that occurs odd times, we need to find that number.
Python Program to Find the Number Occurring Odd Number of Times in a List
def find_odd_occurrence(arr): result = 0 for num in arr: result ^= num return result input_list = list(map(int, input("Enter the list of integers separated by spaces: ").split())) odd_occurrence = find_odd_occurrence(input_list) print("The number occurring odd number of times is: {odd_occurrence}")
How it works
- The program defines a function
find_odd_occurrence
that takes a list of integers as input. - Inside the function, a variable
result
is initialized to 0. We will use this variable to store the XOR of all elements in the list. - We iterate through each number in the list and perform a bitwise XOR operation with the
result
variable. - XOR has a property that if we XOR a number with itself, the result is 0. So, when all even occurrences are XORed, they cancel out and the remaining value is the number occurring an odd number of times.
- The final result is returned by the function.
Input / output
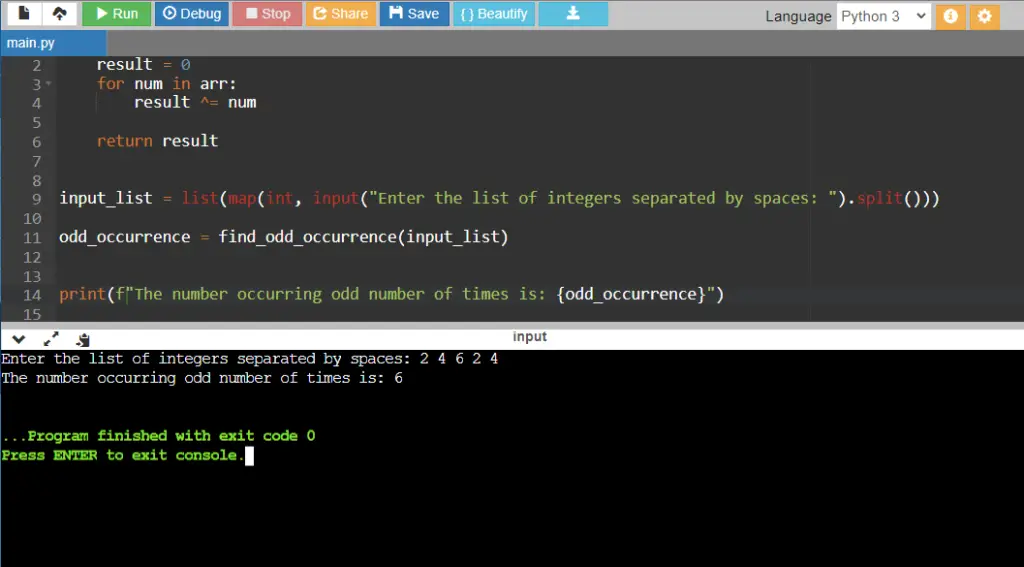