In this Python program, we will convert temperature values from Celsius to Fahrenheit. The Celsius and Fahrenheit scales are two common temperature scales used around the world. Converting Celsius to Fahrenheit involves a simple mathematical formula.
Problem Statement
Given a temperature value in Celsius, we need to convert it to the corresponding value in Fahrenheit.
Python Program to Convert Celsius to Fahrenheit
def celsius_to_fahrenheit(celsius): fahrenheit = (celsius * 9/5) + 32 return fahrenheit # Test the program celsius = float(input("Enter the temperature in Celsius: ")) fahrenheit = celsius_to_fahrenheit(celsius) print(f"Temperature in Fahrenheit: {fahrenheit}°F")
How It Works
- The function
celsius_to_fahrenheit(celsius)
takes a temperature value in Celsius as input and returns the corresponding temperature value in Fahrenheit. - The conversion formula from Celsius to Fahrenheit is:
F = (C * 9/5) + 32
, whereF
represents Fahrenheit andC
represents Celsius. - We apply the formula to the input Celsius value and store the result in the variable
fahrenheit
. - Finally, we return
fahrenheit
, which represents the temperature value in Fahrenheit.
Input/Output
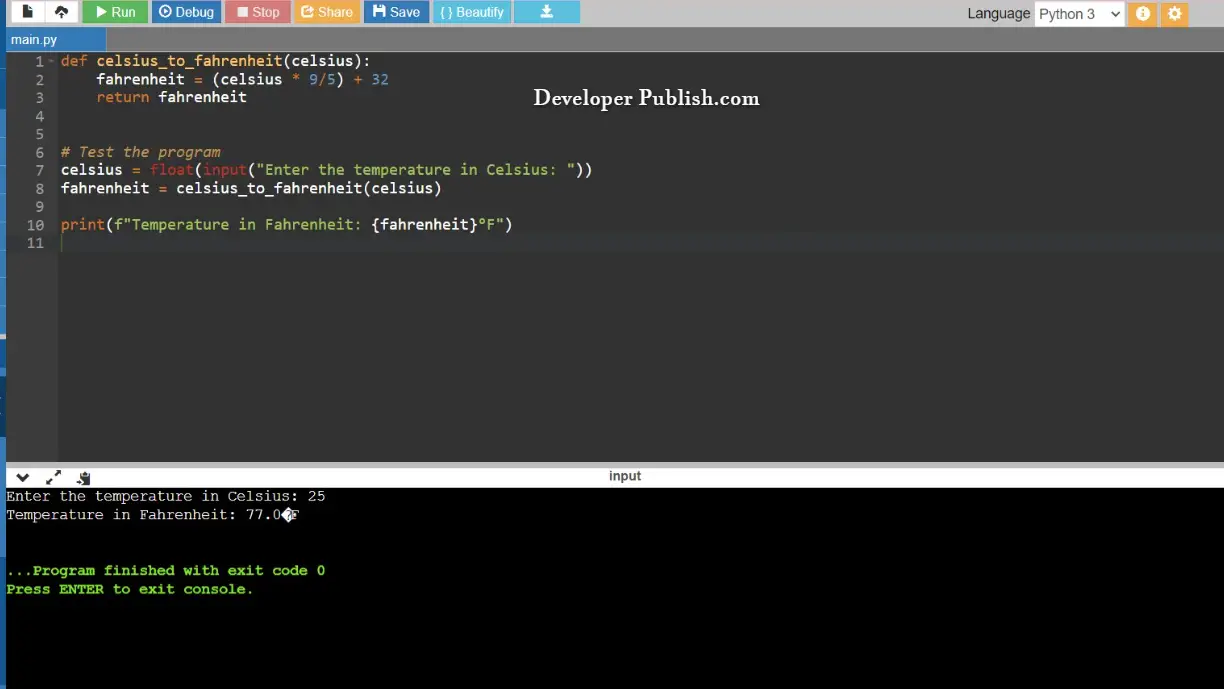