In this Python program, we will create a Python script to check whether a given number is prime or not. A prime number is a positive integer greater than 1 that has no positive divisors other than 1 and itself.
Problem Statement
Given an input number, we need to determine whether it is a prime number or not.
Python Program to Check Prime Number
def is_prime(num): if num <= 1: return False for i in range(2, int(num ** 0.5) + 1): if num % i == 0: return False return True # Main program number = int(input("Enter a number: ")) if is_prime(number): print(number, "is a prime number.") else: print(number, "is not a prime number.")
How it works
- The program defines a function
is_prime(num)
that takes a number as input and checks if it is prime or not. - If the given number
num
is less than or equal to 1, the function returnsFalse
as numbers less than or equal to 1 are not prime. - The function then loops from 2 to the square root of
num
(inclusive) and checks ifnum
is divisible by any of these numbers. - If
num
is divisible by any number in the range, it is not a prime number, and the function returnsFalse
. - If no factors are found, the function returns
True
, indicating that the number is prime. - In the main program, the user is prompted to enter a number. The input is stored in the
number
variable. - The program then calls the
is_prime
function with thenumber
as an argument. - If the returned value is
True
, it means the number is prime, and a corresponding message is printed. - If the returned value is
False
, it means the number is not prime, and a different message is printed.
Input / output
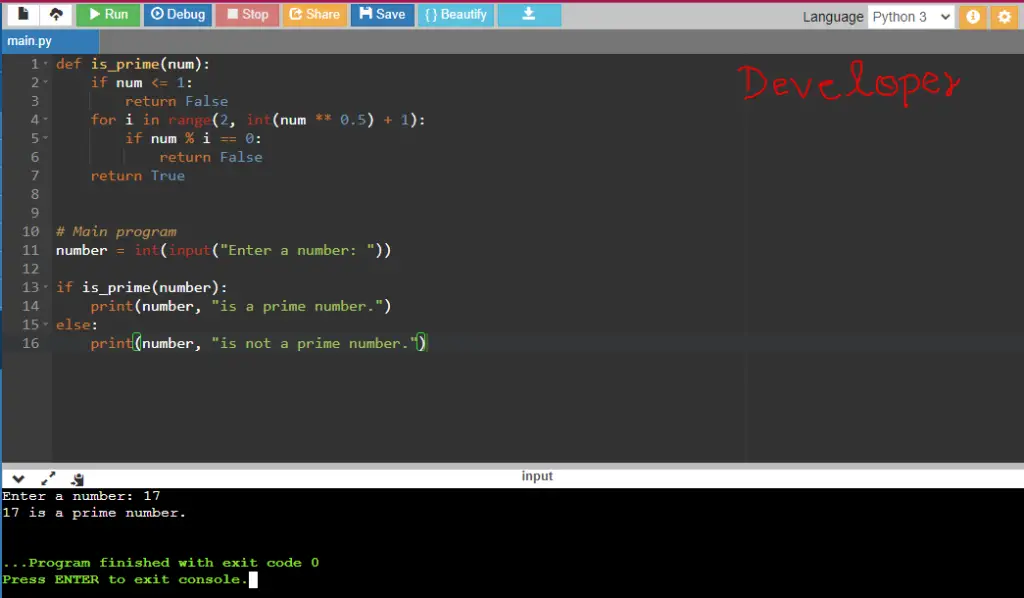