This Python program is designed to copy the contents of one file to another file. It provides a simple and efficient way to duplicate the content from a source file to a destination file. The program handles potential errors such as missing source files or unexpected issues during the copying process.
Problem Statement
By using this program, users can conveniently replicate the content of a file, which can be particularly useful for tasks like data backup or creating duplicates of important documents.
Python Program to Copy One File to Another File
def copy_file(source_file, destination_file): try: with open(source_file, 'r') as source: with open(destination_file, 'w') as destination: for line in source: destination.write(line) print("File copied successfully!") except FileNotFoundError: print("Source file not found.") except Exception as e: print(f"An error occurred: {e}") def main(): print("File Copy Program\n") source_file = input("Enter the source file name: ") destination_file = input("Enter the destination file name: ") copy_file(source_file, destination_file) if __name__ == "__main__": main()
How it works
- The program defines a function
copy_file
that takes two arguments:source_file
anddestination_file
. - Inside the function, it attempts to open the source file in read mode (
'r'
) and the destination file in write mode ('w'
). - It then iterates through each line of the source file and writes it to the destination file.
- The
main
function is defined to interact with the user. It prompts the user to input the source and destination file names. - The
copy_file
function is called with the provided file names. - If the source file is not found, a
FileNotFoundError
is caught and an appropriate message is displayed. Other exceptions are caught using a genericException
and their messages are displayed.
Input / Output
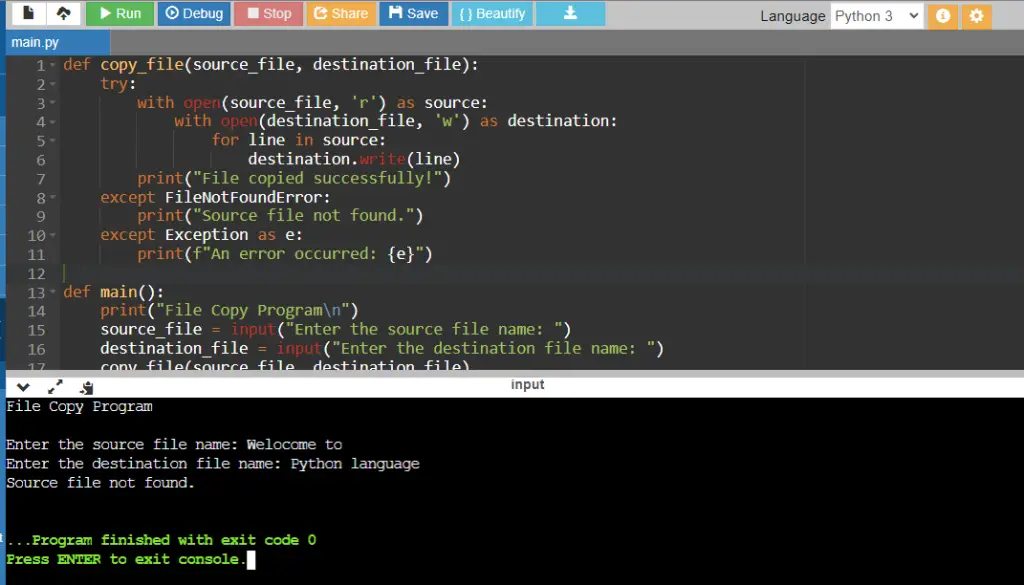