This python program counts the occurrences of a specific letter in a text file.
Problem statement
This program begins with an introduction that explains its purpose. It then takes the path to the text file and the target letter as inputs from the user. The program reads the file, cleans the content by removing punctuation and converting to lowercase, and counts the occurrences of the target letter.
Python Program to Counts the Number of Times a Letter Appears in the Text File
import string file_path = input("\nEnter the path to the text file: ") target_letter = input("Enter the letter to count: ") try: with open(file_path, 'r') as file: content = file.read() cleaned_content = content.translate(str.maketrans('', '', string.punctuation)) cleaned_content = cleaned_content.lower() letter_count = cleaned_content.count(target_letter.lower()) print(f"The letter '{target_letter}' appears {letter_count} times in the text file.") except FileNotFoundError: print("File not found.")
How it works
- The program will prompt you to enter the path to a text file.
- You’ll then input a letter for which you want to count occurrences.
- The program reads the file, processes its content, and counts how many times the given letter appears.
- Finally, the program displays the count of occurrences of the letter in the text file.
Input / Output
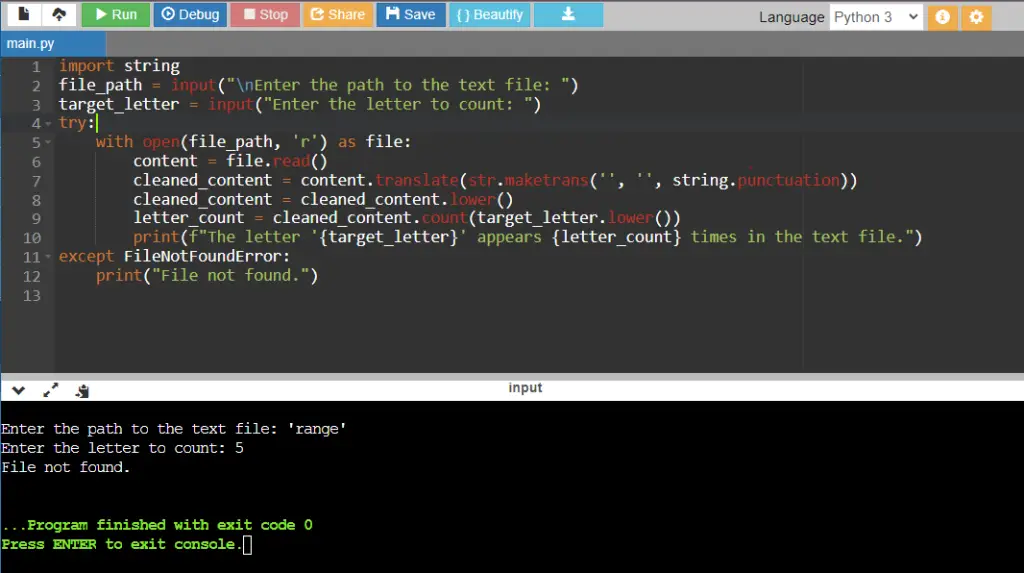