This Python program reads and prints the contents of a file in reverse order.
Printing the contents of a file in reverse order involves reading the lines of the file and presenting them in reverse sequence. This process effectively flips the order of lines from the last line to the first. Python, with its file-handling capabilities and string manipulation functions, makes this task relatively straightforward.
Problem Statement
You are given a task to write a Python program that reads the contents of a text file and prints its contents in reverse order, line by line.
Python Program to Print the Contents of File in Reverse Order
def print_file_in_reverse(file_path): try: with open(file_path, 'r') as file: lines = file.readlines() reversed_lines = reversed(lines) for line in reversed_lines: print(line.strip()) except FileNotFoundError: print("File not found!") # Replace 'file.txt' with the path of the file you want to read in reverse file_path = 'file.txt' print_file_in_reverse(file_path)
How it Works
- Function Definition: This defines a function named
print_file_in_reverse
that takes a single argumentfile_path
, which is the path of the file to be read and printed in reverse order. - Opening the File:This code opens the file specified by
file_path
in read mode ('r'
). It uses thewith
statement, which ensures that the file is properly closed after reading, even if an exception occurs. - Reading Lines: This reads all the lines from the file and stores them in the
lines
list. Each line is stored as a string in the list. - Reversing Lines:The
reversed()
function is used to create an iterator that yields the lines in reverse order. It doesn’t immediately reverse the list but provides a way to iterate over the elements in reverse. - Printing in Reverse:This loop iterates through each line in
reversed_lines
(which contains the lines of the file in reverse order). Thestrip()
method is used to remove any leading or trailing whitespace, including the newline character. Each line is then printed, resulting in the contents of the file being printed in reverse order. - Exception Handling: If the specified file is not found at the given path, a
FileNotFoundError
exception is raised. The program catches this exception and prints an error message indicating that the file was not found.
Input/ Output
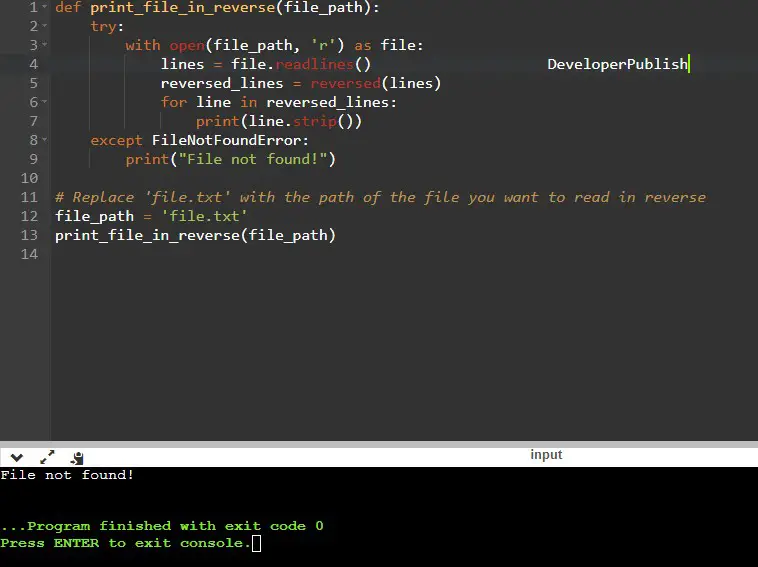