This python program demonstrates the implementation of various doubly linked list operations using a custom class in Python. Doubly linked lists are data structures that allow elements to be connected in both forward and backward directions.
Problem Statement
Implement a doubly linked list class with the following operations:
- Insert an element at the beginning of the list.
- Insert an element at the end of the list.
- Delete an element from the list.
- Display the elements of the list in forward and backward directions.
Program
class Node: def __init__(self, data): self.data = data self.prev = None self.next = None class DoublyLinkedList: def __init__(self): self.head = None def insert_at_beginning(self, data): new_node = Node(data) if not self.head: self.head = new_node else: new_node.next = self.head self.head.prev = new_node self.head = new_node def insert_at_end(self, data): new_node = Node(data) if not self.head: self.head = new_node else: current = self.head while current.next: current = current.next current.next = new_node new_node.prev = current def delete(self, data): if not self.head: return if self.head.data == data: if self.head.next: self.head = self.head.next self.head.prev = None else: self.head = None return current = self.head while current: if current.data == data: if current.next: current.prev.next = current.next current.next.prev = current.prev else: current.prev.next = None return current = current.next def display_forward(self): current = self.head while current: print(current.data, end=" -> ") current = current.next print("None") def display_backward(self): current = self.head while current and current.next: current = current.next while current: print(current.data, end=" -> ") current = current.prev print("None") if __name__ == "__main__": dll = DoublyLinkedList() dll.insert_at_beginning(3) dll.insert_at_beginning(2) dll.insert_at_beginning(1) dll.insert_at_end(4) dll.insert_at_end(5) print("Doubly Linked List (Forward):") dll.display_forward() print("Doubly Linked List (Backward):") dll.display_backward() dll.delete(3) dll.delete(5) print("Doubly Linked List after deletions (Forward):") dll.display_forward()
How it works
The program defines a Node
class to represent elements in the doubly linked list. The DoublyLinkedList
class contains methods to perform various operations like insertion, deletion, and display of elements.
Input / Output
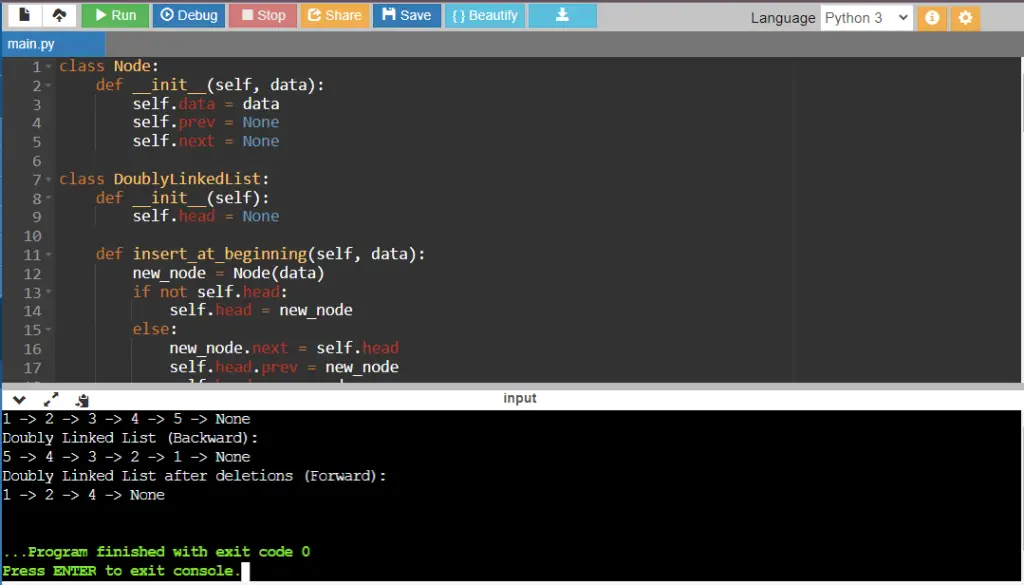