A singly linked list is a data structure that consists of a sequence of nodes, where each node stores an element and a reference (link) to the next node in the sequence. In this python program, we will implement various operations on a singly linked list using Python.
Problem Statement
Create a Python program that performs the following singly linked list operations:
- Insert an element at the beginning of the linked list.
- Insert an element at the end of the linked list.
- Delete an element from the linked list.
- Display the linked list.
Python Program to Perform Singly Linked List Operations
class Node: def __init__(self, data): self.data = data self.next = None class LinkedList: def __init__(self): self.head = None def insert_at_beginning(self, data): new_node = Node(data) new_node.next = self.head self.head = new_node def insert_at_end(self, data): new_node = Node(data) if not self.head: self.head = new_node return current = self.head while current.next: current = current.next current.next = new_node def delete_element(self, target): if not self.head: return if self.head.data == target: self.head = self.head.next return current = self.head while current.next: if current.next.data == target: current.next = current.next.next return current = current.next def display(self): current = self.head while current: print(current.data, end=" -> ") current = current.next print("None") linked_list = LinkedList() linked_list.insert_at_beginning(3) linked_list.insert_at_end(5) linked_list.insert_at_beginning(1) linked_list.insert_at_end(7) linked_list.delete_element(5) print("Linked List:") linked_list.display()
How it works
- The program defines a
Node
class that represents each element in the linked list. - The
LinkedList
class is defined to handle various operations on the linked list. - The
insert_at_beginning
method inserts a new node with the given data at the beginning of the list. - The
insert_at_end
method inserts a new node with the given data at the end of the list. - The
delete_element
method removes the node with the specified data from the list. - The
display
method prints the linked list elements sequentially.
Input / Output
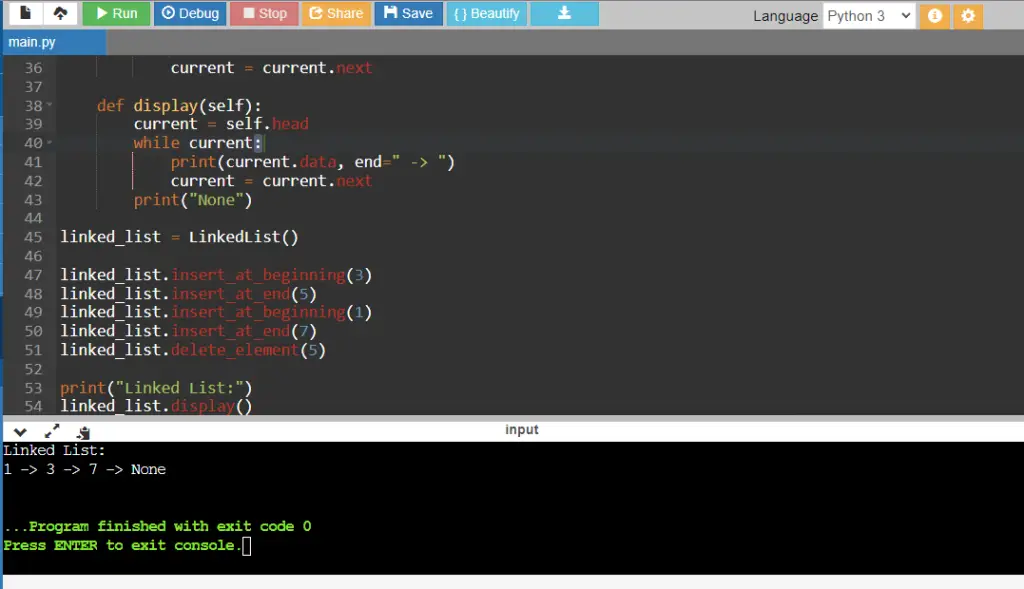