This Python program counts the number of digits and letters in a string. In many text processing applications, it’s often necessary to analyze the contents of a string to determine the number of letters (alphabetic characters) and digits (numeric characters) it contains. This Python program accomplishes this task by taking a user-input string and then counting the occurrences of letters and digits within it.
Problem statement
You are given a string of characters, which may include letters, digits, and other symbols. Your task is to write a Python program that counts the number of digits and letters in this string and displays the results.
Python Program to Count the Number of Digits and Letters in a String
# Function to count the number of digits and letters in a string def count_digits_and_letters(input_string): # Initialize counters for digits and letters num_digits = 0 num_letters = 0 # Iterate through each character in the input string for char in input_string: # Check if the character is a digit if char.isdigit(): num_digits += 1 # Check if the character is a letter elif char.isalpha(): num_letters += 1 return num_digits, num_letters # Input string input_string = input("Enter a string: ") # Call the function and get the results digits, letters = count_digits_and_letters(input_string) # Display the results print("Number of digits:", digits) print("Number of letters:", letters)
How it works
The Python program that counts the number of digits and letters in a string works by iterating through each character in the input string and checking whether each character is a digit or a letter. It maintains two counters: one for counting digits and another for counting letters. Here’s how it works step by step:
- Input: The program takes an input string from the user. This input string can contain a mix of characters, including letters, digits, and other symbols.
- Function Definition: The program defines a function called
count_digits_and_letters(input_string)
to encapsulate the counting logic. This function takes the input string as an argument. - Initialize Counters: Inside the function, two counters,
num_digits
andnum_letters
, are initialized to zero. These counters will keep track of the number of digits and letters encountered in the string. - Iterate through the String: The program then iterates through each character in the input string using a
for
loop. For each character, it does the following:- It checks if the character is a digit using the
isdigit()
method. If it’s a digit, it increments thenum_digits
counter by 1. - It checks if the character is a letter (an alphabetic character) using the
isalpha()
method. If it’s a letter, it increments thenum_letters
counter by 1. - If the character is neither a digit nor a letter, it’s simply ignored; the program doesn’t count it.
- It checks if the character is a digit using the
- Results: After processing all characters in the input string, the program returns the counts of digits and letters.
- Output: Finally, the program prints the results, displaying the number of digits and the number of letters in the input string.
Here’s an example of how it works with the input string “Hello123World”:
- It iterates through each character:
- ‘H’ is a letter, so
num_letters
becomes 1. - ‘e’ is a letter, so
num_letters
becomes 2. - ‘l’ is a letter, so
num_letters
becomes 3. - ‘o’ is a letter, so
num_letters
becomes 4. - ‘1’ is a digit, so
num_digits
becomes 1. - ‘2’ is a digit, so
num_digits
becomes 2. - ‘3’ is a digit, so
num_digits
becomes 3. - ‘W’ is a letter, so
num_letters
becomes 5. - ‘o’ is a letter, so
num_letters
becomes 6. - ‘r’ is a letter, so
num_letters
becomes 7. - ‘l’ is a letter, so
num_letters
becomes 8. - ‘d’ is a letter, so
num_letters
becomes 9.
- ‘H’ is a letter, so
- The program then prints:
- Number of digits: 3
- Number of letters: 9
This demonstrates how the program accurately counts the digits and letters in the input string while ignoring other characters.
Input/Output
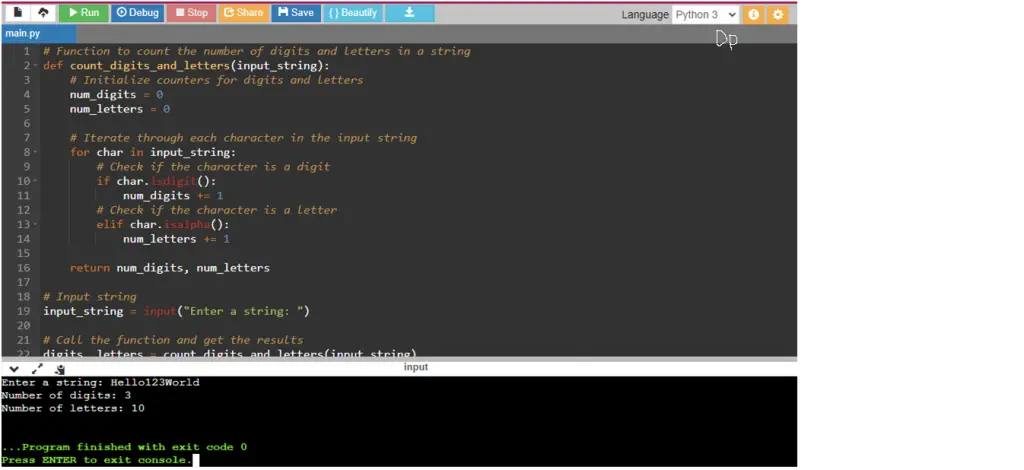