C# provides a convenient way to generate random numbers for various purposes, such as simulations, games, and testing. In this example, we’ll create a C# program to generate random numbers, showcasing the language’s built-in random number generation capabilities.
Program Statement
Write a C# program to generate and display random numbers. The program should generate a specified number of random integers within a given range and display them to the user.
C# Program to Generate Random Numbers
using System; class Program { static void Main() { Console.Write("Enter the number of random numbers to generate: "); int count = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter the minimum value: "); int min = Convert.ToInt32(Console.ReadLine()); Console.Write("Enter the maximum value: "); int max = Convert.ToInt32(Console.ReadLine()); Console.WriteLine("Random numbers generated:"); Random random = new Random(); for (int i = 0; i < count; i++) { int randomNumber = random.Next(min, max + 1); Console.WriteLine(randomNumber); } } }
Input / Output
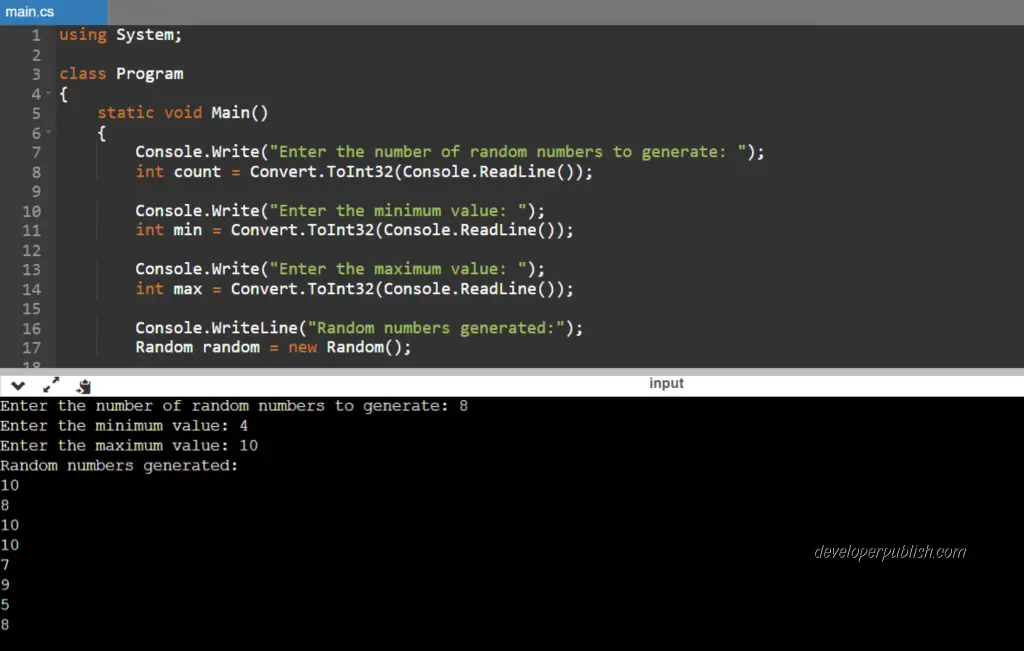