This C# program retrieves and displays the current local time for the system. Local time represents the time in the specific time zone where the computer is located
Problem statement
You are tasked with creating a C# program that retrieves and displays the current local date and time.
C# Program to Get the Local Time
using System; class Program { static void Main() { // Get the current local time DateTime localTime = DateTime.Now; // Print the local time Console.WriteLine("Local Time: " + localTime); } }
How it works
Code: using System;
This line at the beginning of the program is an import statement that allows us to use classes and functions from the System
namespace, which includes the DateTime
struct and the Console
class for input/output operations.
Code:
class Program
{
static void Main()
{
DateTime localTime = DateTime.Now;
Here, we define a class named Program
. Inside this class, we have a method named Main
. This method is the entry point of the program. When you run the program, execution starts here.
DateTime localTime = DateTime.Now;
creates a DateTime
object named localTime
and assigns it the current local date and time. DateTime.Now
returns a DateTime
object representing the current date and time.
Code:
Console.WriteLine("Local Time: " + localTime);
This line prints a message to the console. Console.WriteLine()
is a method that outputs a line of text to the console. In this case, we’re displaying the string “Local Time: ” followed by the value of the localTime
variable.
Code:
}
}
These closing braces (}
) mark the end of the Main
method and the end of the Program
class.
So, when you run this program, it performs the following steps:
- Imports the necessary libraries.
- Defines a class named
Program
. - Defines a method named
Main
which is the starting point of the program. - Inside
Main
, it retrieves the current local date and time and stores it in aDateTime
variable namedlocalTime
. - It then prints a message to the console, including the local date and time.
When you run the program, it will display the current local date and time in the console. The output will be something like:
Code: Local Time: 2023-09-09 15:30:45
Keep in mind that the actual date and time will vary depending on when you run the program.
Input/Output
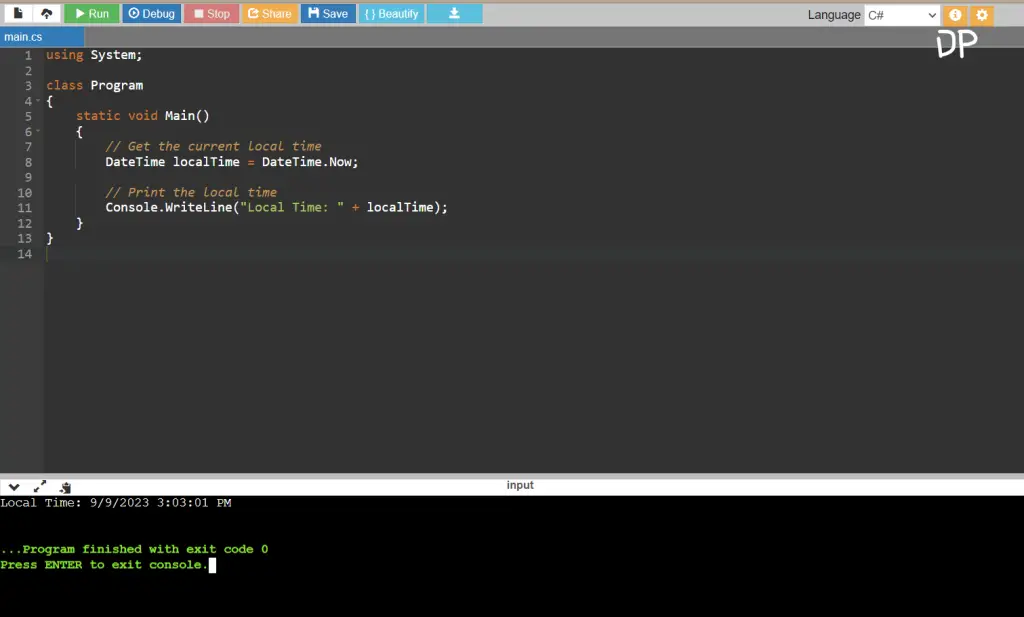