This C# program retrieves and displays the IP (Internet Protocol) address of the computer on which it is executed. The IP address is a unique numerical label assigned to each device on a computer network, allowing them to communicate with one another. Getting an IP (Internet Protocol) address is an essential concept in computer networking and programming. An IP address is a numerical label assigned to each device connected to a computer network that uses the Internet Protocol for communication.
Problem Statement
Write a program in C# that validates whether a given string is a valid IP address. The program should accept both IPv4 and IPv6 addresses and provide feedback on whether the input is valid.
C# Program to Get IP Address
using System; using System.Net; class Program { static void Main() { Console.Write("Enter a host name or IP address: "); string hostNameOrIp = Console.ReadLine(); try { IPAddress[] addresses = Dns.GetHostAddresses(hostNameOrIp); Console.WriteLine($"IP addresses for {hostNameOrIp}:"); foreach (IPAddress address in addresses) { Console.WriteLine(address); } } catch (Exception ex) { Console.WriteLine($"An error occurred: {ex.Message}"); } } }
How it Works
- Choosing a Programming Language:
- You first select a programming language to write your application. In this example, we’ll use C#.
- Import Necessary Libraries or Namespaces:
- Depending on the language, you may need to import specific libraries or namespaces that provide the necessary functions for working with IP addresses. In C#, you need to import the
System.Net
namespace for this purpose.
using System; using System.Net;
- Depending on the language, you may need to import specific libraries or namespaces that provide the necessary functions for working with IP addresses. In C#, you need to import the
- Retrieve IP Address:
- To get an IP address, you use a method or function provided by the language or libraries. In C#, you can use the
Dns.GetHostAddresses()
method, which is part of theSystem.Net
namespace.
- The user is prompted to enter a host name or IP address.
- The
Dns.GetHostAddresses()
method is used to retrieve IP addresses associated with the provided host name or IP address. It returns an array ofIPAddress
objects. - The program then iterates through the array of IP addresses and displays each one to the console.
- To get an IP address, you use a method or function provided by the language or libraries. In C#, you can use the
- Display or Use the IP Address:
- Once you have retrieved the IP address, you can display it to the user or use it for various purposes within your application. In this example, we simply print the IP addresses to the console.
- Error Handling:
- It’s important to include error handling, as network-related operations can encounter issues, such as invalid host names or network problems. In the example, we use a
try-catch
block to catch and handle any exceptions that may occur during the IP address retrieval process.
- It’s important to include error handling, as network-related operations can encounter issues, such as invalid host names or network problems. In the example, we use a
Input/Output
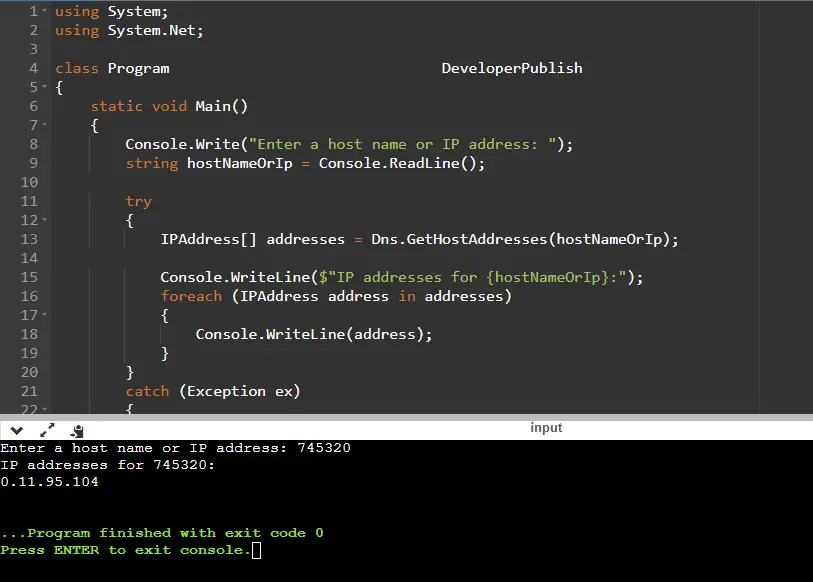