This program is designed to demonstrate how to display the current date in various formats using C#. It showcases different methods available in the DateTime
class to present dates in both standard and custom formats. Understanding date formatting is essential for applications where presenting dates in a user-friendly manner is crucial.
Problem statement
You are tasked with creating a C# program that displays the current date in various formats. The program should utilize different methods available in the DateTime
class to present dates in both standard and custom formats.
C# Program to Display the Date in Various Formats
using System; class Program { static void Main() { DateTime now = DateTime.Now; Console.WriteLine("Current Date in Various Formats:"); Console.WriteLine("1. Long Date: " + now.ToLongDateString()); Console.WriteLine("2. Long Date with Time: " + now.ToLongDateString() + " " + now.ToLongTimeString()); Console.WriteLine("3. Short Date: " + now.ToShortDateString()); Console.WriteLine("4. Short Date with Time: " + now.ToShortDateString() + " " + now.ToShortTimeString()); Console.WriteLine("5. Custom Format (yyyy-MM-dd): " + now.ToString("yyyy-MM-dd")); Console.WriteLine("6. Custom Format (dd-MMM-yy): " + now.ToString("dd-MMM-yy")); } }
How it works
Using Directives:
Code: using System;
- This line includes a directive to use the
System
namespace. This namespace contains fundamental types and base types that define commonly used data types, as well as a variety of utility classes and support classes.
Class Definition:
Code:
class Program
{
static void Main()
{
// …
}
}
This defines a class named Program
. It contains a single method, Main
, which serves as the entry point for the program.
Obtaining Current Date and Time:
Code: DateTime now = DateTime.Now;
This line creates a DateTime
object named now
and assigns it the current date and time using the DateTime.Now
property.
Displaying Dates in Various Formats:
Code: Console.WriteLine(“Current Date in Various Formats:”);
This line prints a header to indicate that the program is about to display dates in various formats.
Code: Console.WriteLine(“1. Long Date: ” + now.ToLongDateString());
This line prints the current date in a long format using the ToLongDateString()
method of the DateTime
class.
Code:
Console.WriteLine(“2. Long Date with Time: ” + now.ToLongDateString() + ” ” + now.ToLongTimeString());
- This line prints the current date and time in a long format using both
methods.
Similarly, the program displays dates in short date formats, along with custom formats (yyyy-MM-dd and dd-MMM-yy).
Running the Program:
When you run the program, it will execute the code within the Main
method. It will get the current date and time, and then display the dates in various formats as specified.
Output:
The program will output the current date in the specified formats, with each format labeled accordingly.
For example, the output may look like this:
Code:
Current Date in Various Formats:
- Long Date: Monday, September 09, 2023
- Long Date with Time: Monday, September 09, 2023 12:34:56 PM
- Short Date: 09/09/2023
- Short Date with Time: 09/09/2023 12:34 PM
- Custom Format (yyyy-MM-dd): 2023-09-09
- Custom Format (dd-MMM-yy): 09-Sep-23
This program demonstrates how to work with dates in C# and how to format them for different purposes using the DateTime
class and its various formatting methods.
Input/Output
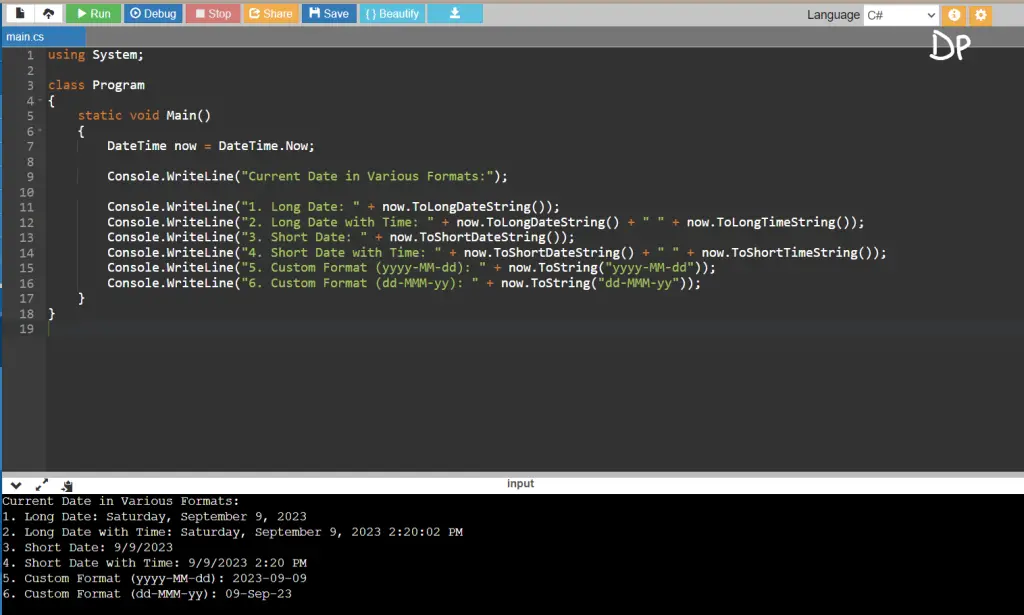