This C# program retrieves and displays the current Coordinated Universal Time (UTC). Coordinated Universal Time, commonly known as UTC, is the primary time standard by which the world regulates clocks and time. It is the same worldwide and does not have any time zone offsets.
Problem statement
You are tasked with creating a C# program that retrieves and displays the current Universal Coordinated Time (UTC). Additionally, the program should extract and display individual components of the UTC time, such as year, month, day, hour, minute, and second.
C# Program to Get the Universal Time
using System; class Program { static void Main() { // Get the current UTC time DateTime utcTime = DateTime.UtcNow; // Print the UTC time in a specific format Console.WriteLine("Current UTC time: " + utcTime.ToString("yyyy-MM-dd HH:mm:ss")); // You can also access individual components of the UTC time if needed int year = utcTime.Year; int month = utcTime.Month; int day = utcTime.Day; int hour = utcTime.Hour; int minute = utcTime.Minute; int second = utcTime.Second; Console.WriteLine($"Year: {year}, Month: {month}, Day: {day}, Hour: {hour}, Minute: {minute}, Second: {second}"); } }
How it works
The C# program provided in the problem statement works by utilizing the DateTime
structure to retrieve and manipulate dates and times.
Here’s a breakdown of how the program works:
Using Directives:
Code: using System;
This line includes the necessary namespaces for the program. In this case, we’re using the System
namespace, which contains fundamental classes and base types.
Class Declaration:
Code:
class Program
{
static void Main()
{
// Code goes here
}
}
This defines a class named Program
. Inside this class, there’s a Main
method which serves as the entry point of the program.
Retrieving UTC Time:
Code: DateTime utcTime = DateTime.UtcNow;
This line retrieves the current UTC time using DateTime.UtcNow
and assigns it to a DateTime
variable named utcTime
.
Displaying UTC Time:
Code: Console.WriteLine(“Current UTC time: ” + utcTime.ToString(“yyyy-MM-dd HH:mm:ss”));
This line prints out the UTC time in a specific format using Console.WriteLine
. The ToString
method is used to format the date and time according to the specified format string ("yyyy-MM-dd HH:mm:ss"
).
Extracting Components:
Code:
int year = utcTime.Year;
int month = utcTime.Month;
int day = utcTime.Day;
int hour = utcTime.Hour;
int minute = utcTime.Minute;
int second = utcTime.Second;
These lines extract individual components of the UTC time using properties of the DateTime
structure (Year
, Month
, Day
, Hour
, Minute
, Second
) and assign them to corresponding variables.
Displaying Component Values:
Code: Console.WriteLine($”Year: {year}, Month: {month}, Day: {day}, Hour: {hour}, Minute: {minute}, Second: {second}”);
This line prints out the extracted components in a formatted string using interpolated strings ($
). The values of the variables year
, month
, day
, etc., are inserted into the string.
Running the Program: When you run this program, it will execute the code inside the Main
method. It will then print out the current UTC time, as well as the individual components of the time.For example, if you run the program at 15:24:30 on September 9, 2023, the output will be similar to:
Code:
Current UTC time: 2023-09-09 15:24:30
Year: 2023, Month: 9, Day: 9, Hour: 15, Minute: 24, Second: 30
That’s how the program works! It retrieves the UTC time, formats and displays it, and also extracts and displays its individual components.
Input/Output
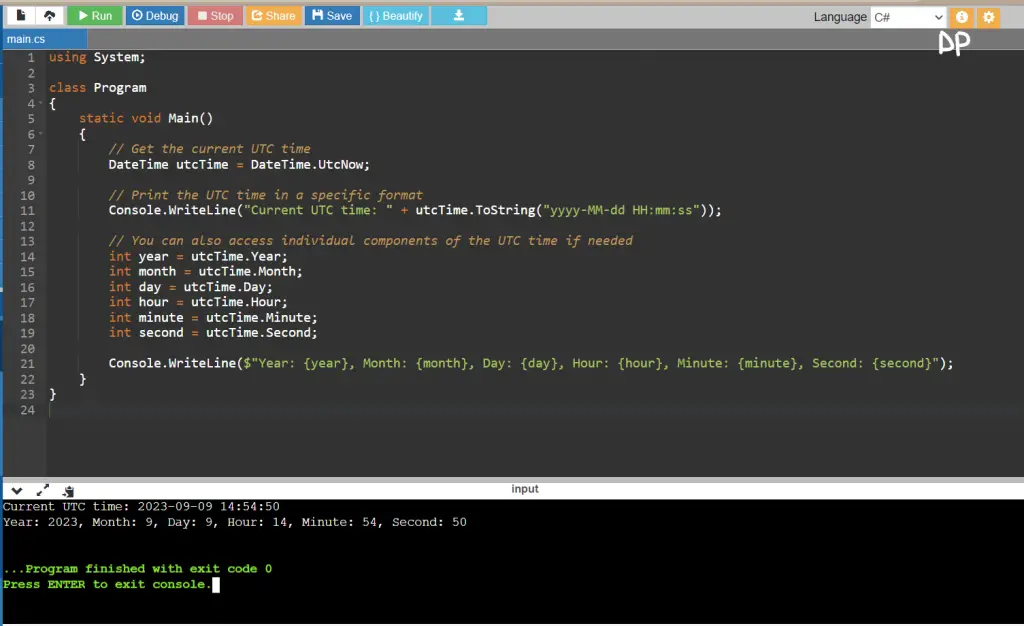