This python program provides an introduction to the user, explaining what it does. It then prompts the user to enter a string, creates a new string based on the first and last two characters using the create_new_string
function, and displays the result. If the input string has less than 2 characters, it informs the user that it’s too short to create a new string.
Problem Statement
You are tasked with creating a Python program that takes an input string and generates a new string consisting of the first two characters and the last two characters of the input string. If the input string has less than two characters, the program should return an empty string.
Python Program to Create a New String Made up of First and Last 2 Characters
# Introduction print("Welcome to the First and Last 2 Characters String Maker!") # Function to create a new string from the first and last two characters of a given string def create_new_string(input_string): # Check if the input string has at least 2 characters if len(input_string) >= 2: # Concatenate the first two characters and the last two characters new_string = input_string[:2] + input_string[-2:] return new_string else: # If the input string has less than 2 characters, return an empty string return "" # Get user input input_string = input("Enter a string: ") # Call the function and display the result result = create_new_string(input_string) if result: print("New string:", result) else: print("The input string is too short to create a new string.")
How it works
The “First and Last 2 Characters String Maker” program works by taking an input string, processing it, and then generating a new string based on the first and last two characters of the input string. Here’s how the program works step by step:
- Introduction: The program starts with a welcome message to introduce itself to the user. This message informs the user about the program’s purpose.
- Function Definition: The program defines a function called
create_new_string(input_string)
. This function takes an input string as a parameter. create_new_string
Function:- The function checks if the input string has at least 2 characters using the
len(input_string)
function. - If the input string has at least 2 characters:
- It extracts the first two characters using slicing with
input_string[:2]
. - It extracts the last two characters using slicing with
input_string[-2:]
. - It concatenates the first two characters and the last two characters to create a new string.
- It returns the new string.
- It extracts the first two characters using slicing with
- If the input string has less than 2 characters, it returns an empty string.
- The function checks if the input string has at least 2 characters using the
- User Input: The program prompts the user to enter a string using
input()
. The user can type any string they want. - Function Call: The program calls the
create_new_string
function and passes the user’s input string as an argument. This is done with the lineresult = create_new_string(input_string)
. - Result Display: The program then checks the result from the
create_new_string
function:- If the result is not an empty string (i.e., the input string had at least 2 characters), it displays the new string using
print("New string:", result)
. - If the result is an empty string (i.e., the input string had less than 2 characters), it displays a message indicating that the input string is too short to create a new string.
- If the result is not an empty string (i.e., the input string had at least 2 characters), it displays the new string using
- Program Completion: The program execution completes, and the user can choose to run it again or exit.
Here’s a summary of what happens when you run the program:
- You see a welcome message.
- You input a string.
- The program processes the string, and if it has at least 2 characters, it creates a new string from the first and last two characters and displays it.
- If the input string has less than 2 characters, it informs you that it’s too short to create a new string.
This program demonstrates how to use functions, string slicing, and conditional statements in Python to manipulate strings based on specific criteria.
Input/Output
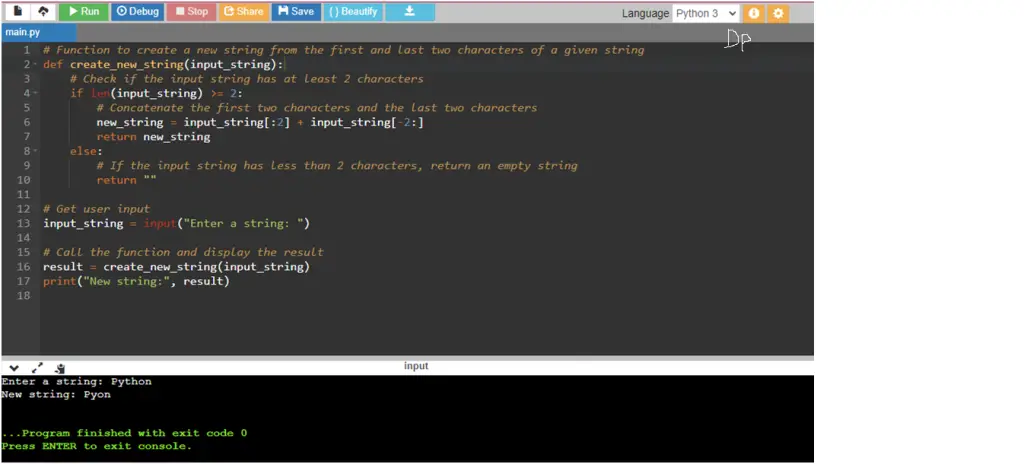