C# Compiler Error
CS0431 – Cannot use alias ‘identifier’ with ‘::’ since the alias references a type. Use ‘.’ instead
Reason for the Error
You’ll get this error in your C# code when you have used :: with an alias while referencing a type.
For example, lets try to compile the below code snippet.
using System; namespace DeveloperPublishNamespace { using A = Class1; public class Class1 { public class Class2 { public static void Function1() { Console.WriteLine("Inside Function1"); } } } class Program { static void Main(string[] args) { A::Class2.Function1(); Console.WriteLine("Hello World!"); } } }
You’ll receive the error code CS0431 when you try to compile the above C# program because we have defined the alias “A” for the class Class1 the nested class’s Function1 is called using the alias A and the :: operator.
Error CS0431 Cannot use alias ‘A’ with ‘::’ since the alias references a type. Use ‘.’ instead. DeveloperPublish C:\Users\Senthil\source\repos\ConsoleApp4\ConsoleApp4\Program.cs 20 Active
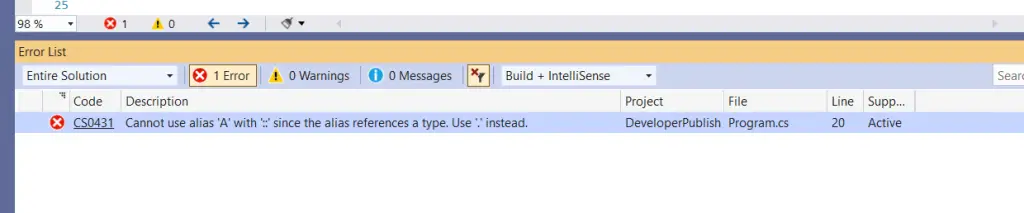
Solution
You can fix this error in your C# program by using the “.” operator instead of “::” operator as shown below.
using System; namespace DeveloperPublishNamespace { using A = Class1; public class Class1 { public class Class2 { public static void Function1() { Console.WriteLine("Inside Function1"); } } } class Program { static void Main(string[] args) { A.Class2.Function1(); Console.WriteLine("Hello World!"); } } }