C# Compiler Error
CS0182 – An attribute argument must be a constant expression, typeof expression or array creation expression of an attribute parameter type
Reason for the Error
There are certain restrictions in C# that apply to the type of parameters that can be used with the attributes. You cannot use sbyte, ushort, uint, ulong, decimal as arguments for the attributes.
You will receive the error CS0182 when you assign a non- constant expression or a parameter of type object to the attributes.
For example, lets try to compile the below code snippet.
namespace DeveloperPubNamespace { using System; class StrAttribute : Attribute { public StrAttribute(string value) { } } class Program { static string input = "DeveloperPublish"; [System.Diagnostics.ConditionalAttribute(input)] void Function1() { } static void Main(string[] args) { } } }
The above code snippet will result with the error code CS0182 because we used the variable input which is a non-constant type.
Error CS0182 An attribute argument must be a constant expression, typeof expression or array creation expression of an attribute parameter type ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 13 Active
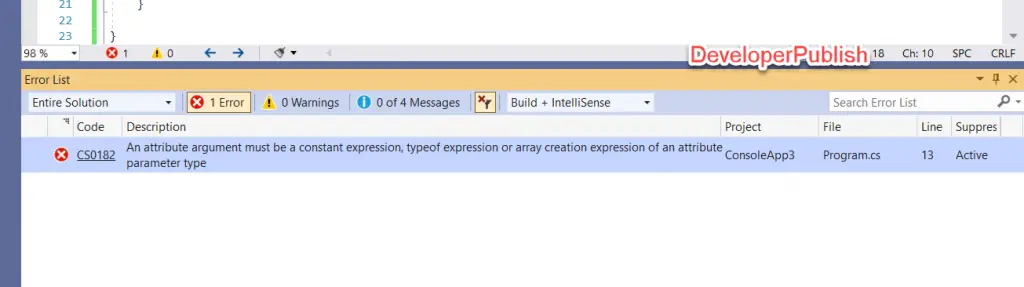
Solution
To fix the error, ensure that the constant expression is used as parameter for the attributes. The above code can be fixed by making the variable input as constant.
namespace DeveloperPubNamespace { using System; class StrAttribute : Attribute { public StrAttribute(string value) { } } class Program { const string input = "DeveloperPublish"; [System.Diagnostics.ConditionalAttribute(input)] void Function1() { } static void Main(string[] args) { } } }