C# Compiler Error
CS0185 – ‘type’ is not a reference type as required by the lock statement
Reason for the Error
You will receive this error in C# when you attempt to use a value type in a lock statement. The lock statement in C# can be used with reference types and NOT value types.
For example, lets try to compile the below code snippet.
namespace DeveloperPubNamespace { public class Class1 { } class Program { static void Main(string[] args) { int input = 10; lock (input) ; } } }
The above code snippet will result with the error code CS0185 because we used the type “int” which is a value type is used with the lock statement.
Error CS0185 ‘int’ is not a reference type as required by the lock statement ConsoleApp3 C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 12 Active
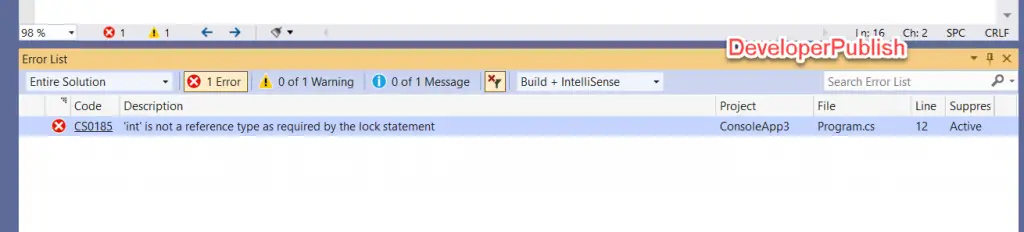
Solution
To fix the error, ensure that you use the reference types instead of value types when using lock statement. For example, the below code would compile without any errors when used with the lock statement.
namespace DeveloperPubNamespace { public class Class1 { } class Program { static void Main(string[] args) { Class1 obj = new Class1(); lock (obj) ; } } }