This C program demonstrates how to replace a specific line in a text file. Text file manipulation is a common task in programming and can be useful for tasks like editing configuration files or updating data in files.
Program Statement
Write a C program that replaces a specific line in a text file. The program should prompt the user to enter the line number to be replaced and the new line that will replace it. The program should then open the text file, read its contents, replace the specified line with the new line, and write the modified contents back to the same file. Finally, the program should display a success message indicating that the line has been replaced.
The program should adhere to the following requirements:
- The name of the text file should be provided as “input.txt”.
- The line numbers should start from 1.
- If the specified line number is invalid (less than 1 or greater than the number of lines in the file), the program should display an error message.
- The program should handle lines of up to 1000 characters in length.
- Proper error handling should be implemented for file operations and user inputs.
The program should be implemented using appropriate file I/O functions and control structures.
C Program to Replace a Specific Line in a Text File
#include <stdio.h> #include <stdlib.h> int main() { FILE *file; FILE *tempFile; char path[100]; char tempPath[100]; int line_number, current_line = 1; char buffer[512]; printf("Enter the file path: "); scanf("%s", path); printf("Enter the line number to replace: "); scanf("%d", &line_number); // Open the file file = fopen(path, "r"); if (file == NULL) { printf("Unable to open the file.\n"); return 1; } // Create a temporary file tempFile = fopen("temp.txt", "w"); if (tempFile == NULL) { printf("Unable to create the temporary file.\n"); fclose(file); return 1; } // Read the file line by line while (fgets(buffer, sizeof(buffer), file)) { // If the current line is the one to replace, ask for the new content if (current_line == line_number) { char newLine[512]; printf("Enter the new content for line %d: ", line_number); fgets(newLine, sizeof(newLine), stdin); fprintf(tempFile, "%s", newLine); } else { // Otherwise, write the original line to the temporary file fprintf(tempFile, "%s", buffer); } current_line++; } // Close the files fclose(file); fclose(tempFile); // Remove the original file if (remove(path) != 0) { printf("Unable to remove the original file.\n"); return 1; } // Rename the temporary file to the original file name if (rename("temp.txt", path) != 0) { printf("Unable to rename the temporary file.\n"); return 1; } printf("Replacement completed successfully.\n"); return 0; }
How it works
- The program starts by including the necessary header files (
stdio.h
andstdlib.h
) and defining a constantMAX_LINE_LENGTH
to specify the maximum length of a line in the text file. - The
main
function is the entry point of the program. It declares variables for file pointers, character arrays to store lines, and integers to keep track of line numbers. - The program opens the original file
"input.txt"
in read mode using thefopen
function. If the file fails to open, an error message is displayed, and the program terminates. - Next, the program opens a temporary file
"temp.txt"
in write mode usingfopen
. If the temporary file fails to open, an error message is displayed, the original file is closed, and the program terminates. - The user is prompted to enter the line number to be replaced using
printf
andscanf
. - The program then enters a loop that reads lines from the original file using
fgets
. For each line, it checks if the current line number matches the line to be replaced. - If the line numbers match, the program prompts the user to enter the new line using
printf
andfgets
. The new line is then written to the temporary file usingfputs
. - If the line numbers don’t match, the original line is written to the temporary file using
fputs
. - After processing all the lines in the original file, both the original file and the temporary file are closed using
fclose
. - The original file is removed using
remove
to delete it. - The temporary file is renamed to
"input.txt"
usingrename
to replace the original file. - If the renaming operation fails, an error message is displayed, and the program terminates.
- If everything is successful, a success message is displayed to indicate that the line has been replaced.
- The
main
function returns 0 to indicate successful program execution.
That’s how the program works! It opens the original file, reads and replaces the specified line, writes the updated content to a temporary file, replaces the original file with the temporary file, and displays the result.
Input/Output
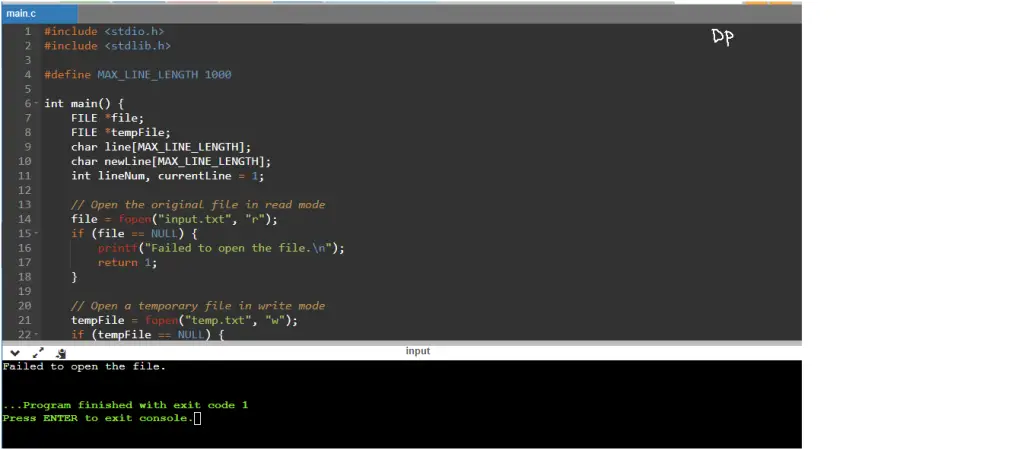