C# Compiler Error
CS0238 – ‘member’ cannot be sealed because it is not an override
Reason for the Error
You will receive this error in your C# program when you have used the sealed keyword for a method without override,
sealed keyword on a class prevents other classes from inheriting from it. The sealed modifier on a method can be used on a method that overrides a virtual method in a base class.
For example, try to compile the below code snippet.
namespace DeveloperPubNamespace { abstract class BaseEmployee { public abstract void SetId(); } class Employee : BaseEmployee { public sealed void SetId() { } } class Program { public static void Main() { } } }
This program will result with the C# error code CS0238 because the SetId() is marked as sealed without override.
Error CS0238 ‘Employee.SetId()’ cannot be sealed because it is not an override DeveloperPublish C:\Users\SenthilBalu\source\repos\ConsoleApp3\ConsoleApp3\Program.cs 9 Active
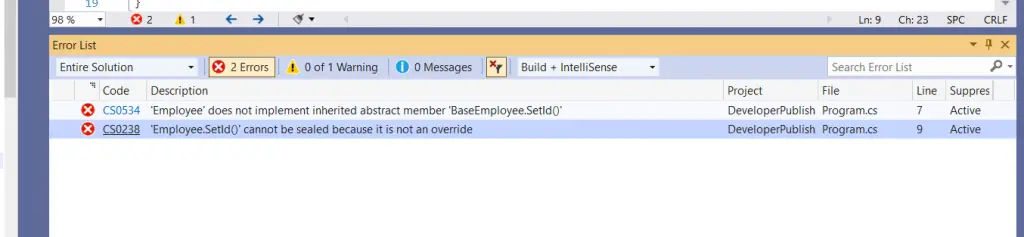
Solution
To fix the error code CS0238 in C#, you should mark the method as sealed override instead of just sealed.
namespace DeveloperPubNamespace { abstract class BaseEmployee { public abstract void SetId(); } class Employee : BaseEmployee { public sealed override void SetId() { } } class Program { public static void Main() { } } }