This blog post will explain one of the new feature called Records that was introduced in C# 9.0. Records in C# 9 makes the entire object immutable where as the similar feature called Init-only properties provides the developers to make the individual properties immutable. Init-Only properties in C# 9.0 is covered in one my previous blog post.
What does the Record Keyword in C# 9 do ?
C# 9.0 introduces a new keyword called record. The record keyword in C# 9 lets the developers to define the object as immutable to behave it like a value type..
Note : The declaration of the records in the earlier preview version was using the data class syntax. Since C# 9.0 is still in preview, it has now changed to record. Initially the data class was considered because the compiler team was considering struct class support.
Here’s a simple example showing how to create a Employee record using the init-properties.
public record Employee { public string FirstName { get; init; } public string LastName { get; init; } public string Designation { get; init; } }
Note that if you need to make the whole object immutable , then you will have to set the init keyword for each property explicitly. Additionally , the members of the Record type are public by default.
With Expression and Records in C# 9.0
In the previous versions of C# 9.0 , if you want to represent a new state of a object , you will likely be copying the values from the old object and modifying it. Unfortunately your object is immutable in this case and the with keyword in C# 9 helps you with it.
For example , assume that you want to change the designation of the existing employee , you will be doing something like this.
var emp = new Employee { FirstName = "Senthil", LastName = "Balu", Designation = "Technical Architect" }; var newDesig = emp; newDesig.Designation = "Solution Architect";
The with keyword in C# allows you to create an object form another one by just specifying the property changes. FOr example , we know that only the Designation is changed in the new object and we specify that value using the with keyword in C# 9. Under the hood , with keyword uses the protected copy constructor.
var emp = new Employee { FirstName = "Senthil", LastName = "Balu", Designation = "Technical Architect" }; var newDesig = emp with { Designation = "Solution Architect" };
Note that as of now , with keyword is only available only for record types. They are not available for structs and class.
Record in C# 9.0 and inheritance
One of the other features of the record is the support of inheritence. The records have a hidden virtual clone method that copies the entire object and with the support of the with keyword , when you assign a child instance to the parent , the content is still retained.
using System; namespace ConsoleApp6 { class Program { static void Main(string[] args) { Employee emp = new EmployeeSalary { FirstName = "Senthil", LastName = "Balu", Designation = "Technical Architect", Basic = 25000 }; var newDesig = emp with { FirstName = "Michael" , LastName = "Bevan" }; // newDesig is of type EmployeeSalary Console.WriteLine(newDesig.GetType()); Console.WriteLine(emp.Designation); Console.WriteLine(newDesig.Designation); Console.ReadLine(); } } public record Employee { public string FirstName { get; init; } public string LastName { get; init; } public string Designation { get; init; } } public record EmployeeSalary : Employee { public int Basic { get; init; } } }
Positional Records in C# 9.0
The positional records brings in the feature of allowing the developers to pass the data via constructor arguments. Yes, constructors and destructors are supported in C# 9.0.
Here’s an example of how to use Positional Records in C#
// Positional Records public record Employee(string FirstName,string LastName,string Designation);
Below is a screenshot showing how you can call the positional records.
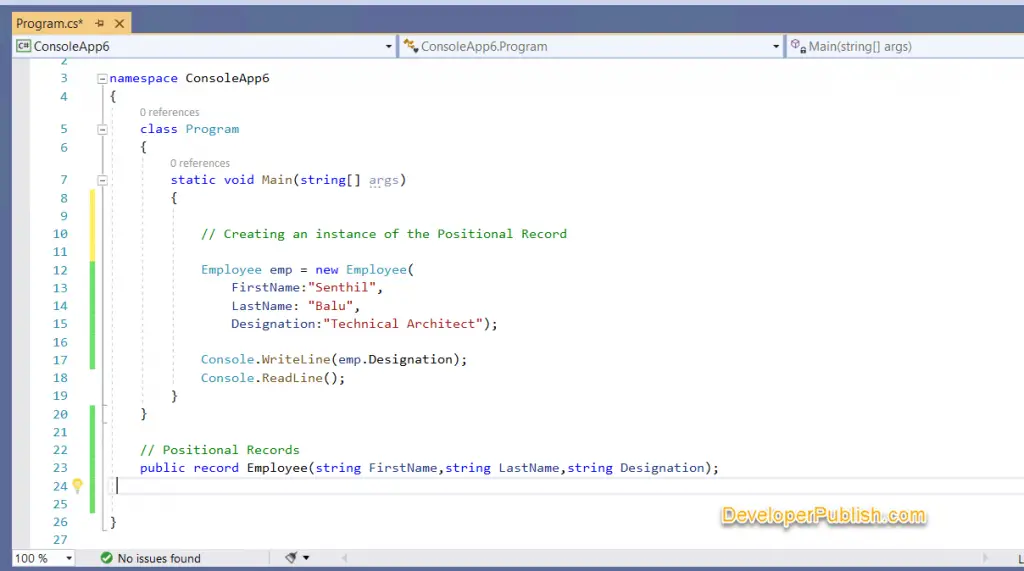
2 Comments
Excellent blog from the ex – Microsoft MVP 🙂
Thank you 🙂