Assume that you have a string in C# and you want to have a mixture of both the upper case and the lowercase (i meant TitleCase in this example).
You can do that easily using the ToTitleCase method defined in the TextInfo class.
How to Convert a string to Title Case in C# ?
Here’s a sample code snippet demonstrating how to do it.
string title = "senthil is a author at developerpublish.com"; System.Globalization.TextInfo textInfo = new System.Globalization.CultureInfo("en-US", false).TextInfo; title = textInfo.ToTitleCase(title); Console.WriteLine(title);
Output
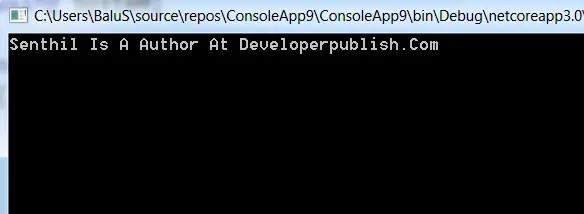
Note that when the text is all uppercase , it would not work as expected. Instead , you could convert the input string to lower case and then convert it in to titlecase.
string title = "SENTHIL IS THE AUTHOR OF DEVELOPERPUBLISH.COM"; System.Globalization.TextInfo textInfo = new System.Globalization.CultureInfo("en-US", false).TextInfo; title = textInfo.ToTitleCase(title.ToLower()); Console.WriteLine(title);