Problem:
You want to convert a simple JSON string to a C# and vice versa object using Json.NET.
Solution
Use the JsonConvert class that is available as part of Json.NET library. The JsconConvert provides the SerializeObject and DeserializeObject functions to perform the simple serialization and deserialization.
Discussion
Assume that you have a customer object with the below properties filled and you wish to convert it to a JSON string, you can use the SerializeObject defined in the JsonConvert class.
public class Customer { public string EmailAddress { get; set; } public DateTime CreatedDate { get; set; } public bool PaymentMade { get; set; } public List<int> OrderIds { get; set; } } Customer customer = new Customer(); customer.EmailAddress = "[email protected]"; customer.CreatedDate = new DateTime(2008, 12, 28); customer.PaymentMade = true; customer.OrderIds = new List<int>() { 7013,7066,7812 }; string output = JsonConvert.SerializeObject(customer);
The output variable would contain the Json string.
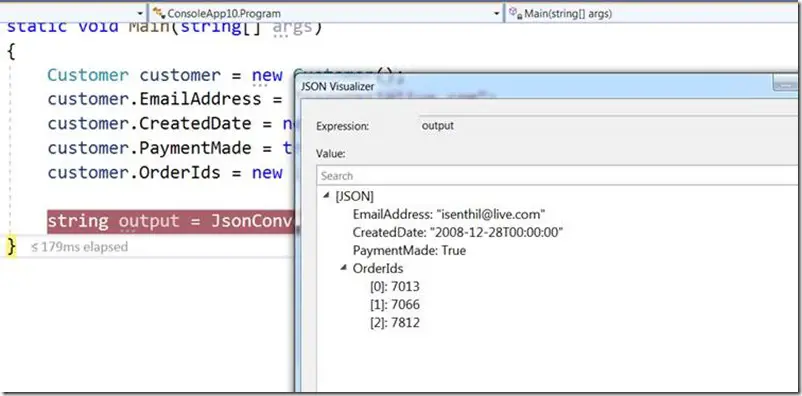
Similarly, if you want to deserialilze the Json string to the C# object, you can use the DeserializeObject method by specified the type to which the Json string needs to be deserialilze as shown below.
string jsonString = "\"{\"EmailAddress\\\":\\\"[email protected]\\\", \\\"CreatedDate\\\":\\\"2008-12-28T00:00:00\\\",\\\"PaymentMade\\\" :true,\\\"OrderIds\\\":[7013,7066,7812]}\""; Customer output = JsonConvert.DeserializeObject<Customer>(jsonString);
If you have a mismatch in the json property name or C# class properties, you would end up getting the Newtonsoft.Json.JsonSerializationException.
For example, if the jsonString contains EmailAddress1 as the property and you have EmailAddress as property in your C# class. When you deserialize, you will receive the below error.
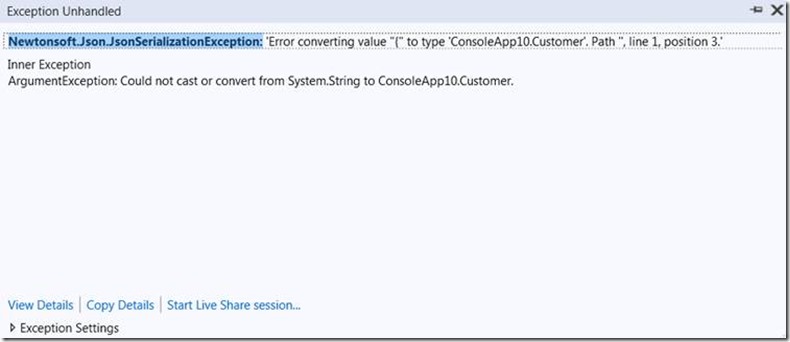