This blog post will explain the feature Init-only properties in C# 9.0 and how to use it.
Object Initializers is of the cool features in the previous versions of C#. It allows the C# developers to create an object and initialize it in a better way. It just frees up the developers from writing a lot of constructor boilerplate code.
Assume that you have a class Person as shown below.
public class Employee { public string FirstName { get; set; } public string LastName { get; set; } }
You create an instance of Employee with the object initializer like this
Employee emp = new Employee { FirstName = "Senthil", LastName = "Balu" };
While this is a great feature , Object Initailizers does have its own limitations. The properties with-in the class has to be mutable for the object initializers to work correctly. When you use object initilizers , it first calls the object’s default constructor without any parameters and then assign the values to the properties using the setters.
Here’s comes Init-only properties in C# 9.0 to fix that issue for you.
Init-only properties in C# 9.0
C# 9.0 introduces a new feature called init accessor which is an kind of variants of set accessor in C# and which can be used only during the object initializers.
public class Employee { public string FirstName { get; init; } public string LastName { get; init; } }
With the new init-only properties , you can still initialize your object using object initializers as shown above. But when you perform any assignment of value to these object properties apart from the initializers , it will result in the compiler error.
“Error CS8852 Init-only property or indexer ‘Employee.FirstName’ can only be assigned in an object initializer, or on ‘this’ or ‘base’ in an instance constructor or an ‘init’ accessor.“
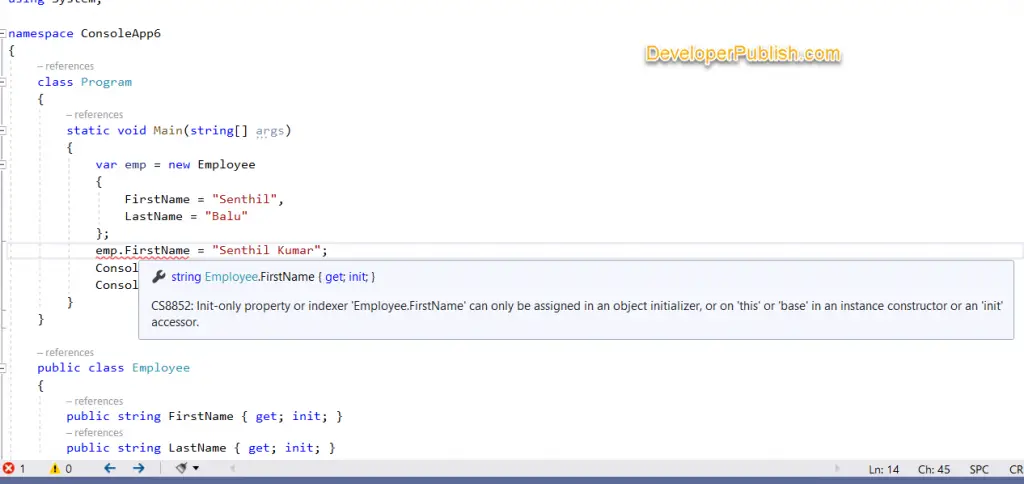
Note : Since init only properties can be called during the object initialization , you can mutate the readonly fields along with too.