In geometry, Pythagoras theorem is used to find the length of the hypotenuse of a right-angled triangle. In this task, we will write a C# program to find a number using Pythagoras theorem.
Problem Statement
Write a C# program to find a number using Pythagoras theorem. The program should take input from the user in the form of the length of the two sides of a right-angled triangle. The program should display the length of the hypotenuse as output.
C# Program to Find a Number using Pythagoras Theorem
using System; class Program { static void Main(string[] args) { double a, b, c; Console.WriteLine("Enter the First Value "); a = double.Parse(Console.ReadLine()); Console.WriteLine("Enter the Second Value "); b = double.Parse(Console.ReadLine()); c = Math.Sqrt(a * a + b * b); Console.WriteLine("The Other Number is : {0}", c); Console.ReadLine(); } }
Input / Output
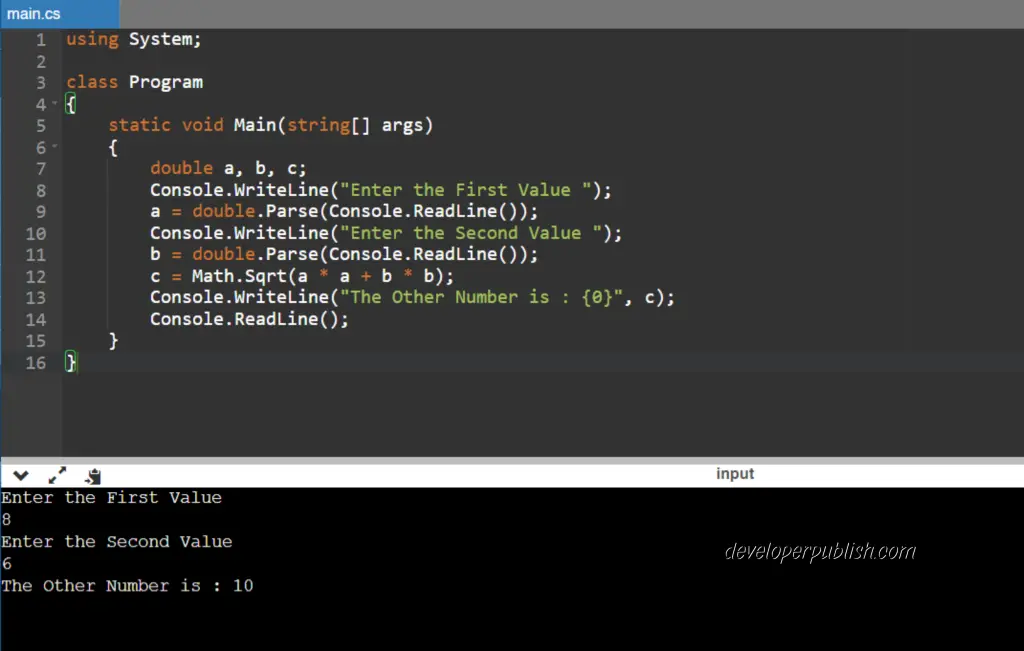