This C# program is designed to count the occurrences of the digit ‘1’ in a user-entered number. It leverages basic programming constructs such as loops and conditional statements to achieve this task.
Problem statement
Write a C# program that counts the number of occurrences of the digit ‘1’ in a user-entered integer.
C# Program to Count the Number of 1’s in the Entered Number
using System; class Program { static void Main() { Console.Write("Enter a number: "); int number = int.Parse(Console.ReadLine()); int count = CountOnes(number); Console.WriteLine($"The number of 1's in the entered number is: {count}"); } static int CountOnes(int num) { int count = 0; while (num > 0) { if (num % 10 == 1) { count++; } num /= 10; } return count; } }
How it works
- User Input:
- The program starts by prompting the user to enter a number using the
Console.Write
method.
- The program starts by prompting the user to enter a number using the
- Reading and Converting Input:
- The entered input is read as a string using
Console.ReadLine()
. - The
int.Parse()
method is used to convert the input string to an integer. This ensures that the program works with numerical values.
- The entered input is read as a string using
- Calling the
CountOnes
Function:- The program then calls a function named
CountOnes
and passes the entered number as an argument.
- The program then calls a function named
CountOnes
Function:- This function is responsible for counting the number of ‘1’s in the entered number.
- Looping Through Digits:
- Inside
CountOnes
, awhile
loop is used. This loop allows the program to go through each digit of the entered number.
- Inside
- Checking for ‘1’:
- In each iteration of the loop, the program checks if the current digit (obtained using
num % 10
) is equal to 1. - If it is, the counter variable
count
is incremented.
- In each iteration of the loop, the program checks if the current digit (obtained using
- Updating the Number:
- The number is then divided by 10 (
num /= 10
). This removes the last digit and allows the loop to continue with the next digit.
- The number is then divided by 10 (
- Returning the Count:
- Once all digits have been processed, the
count
variable holds the total count of ‘1’s, which is returned by the function.
- Once all digits have been processed, the
- Displaying the Result:
- Finally, the program prints out the count of ‘1’s in the entered number using
Console.WriteLine
.
- Finally, the program prints out the count of ‘1’s in the entered number using
- Error Handling:
- If the user enters a non-integer or a negative number, the program would throw an exception during the
int.Parse()
step, which could be caught for handling.
Overall, this program effectively counts the number of occurrences of the digit ‘1’ in the entered number by iteratively examining each digit. It showcases the use of loops, conditional statements, and function usage in C# programming.
Input/Output
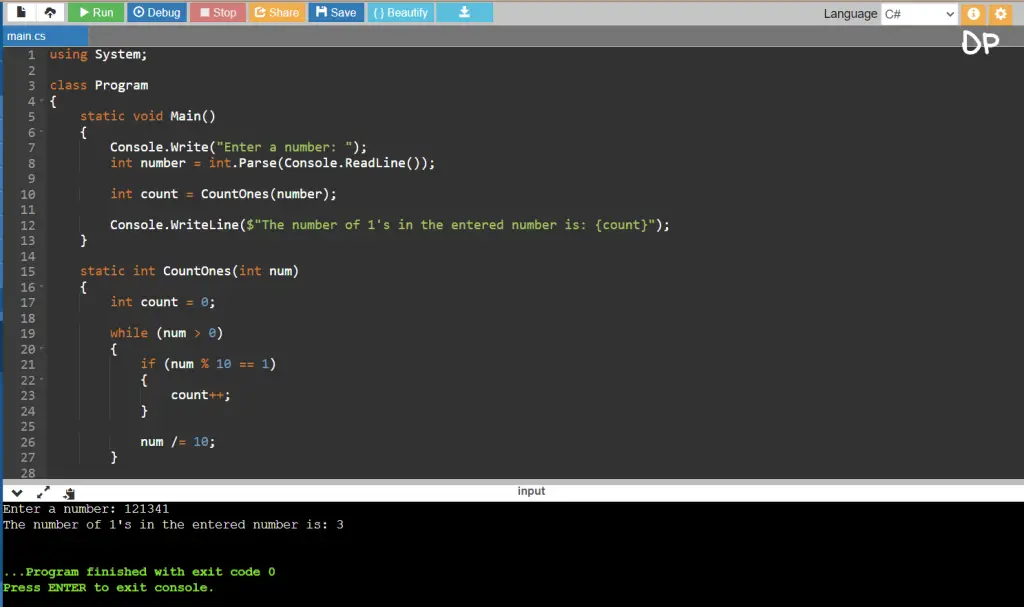