In mathematics, nPr represents the number of permutations of n objects taken r at a time. In this task, we will write a C# program to find nPr.
Problem Statement
Write a C# program to find nPr. The program should take input from the user in the form of the values of n and r. The program should display the value of nPr as output.
C# Program to Find nPr
using System; class Program { static void Main(string[] args) { Console.WriteLine("Enter the value of n: "); int n = int.Parse(Console.ReadLine()); Console.WriteLine("Enter the value of r: "); int r = int.Parse(Console.ReadLine()); int nPr = 1; for (int i = n; i > n - r; i--) { nPr *= i; } Console.WriteLine("nPr: {0}", nPr); } }
Input / Output
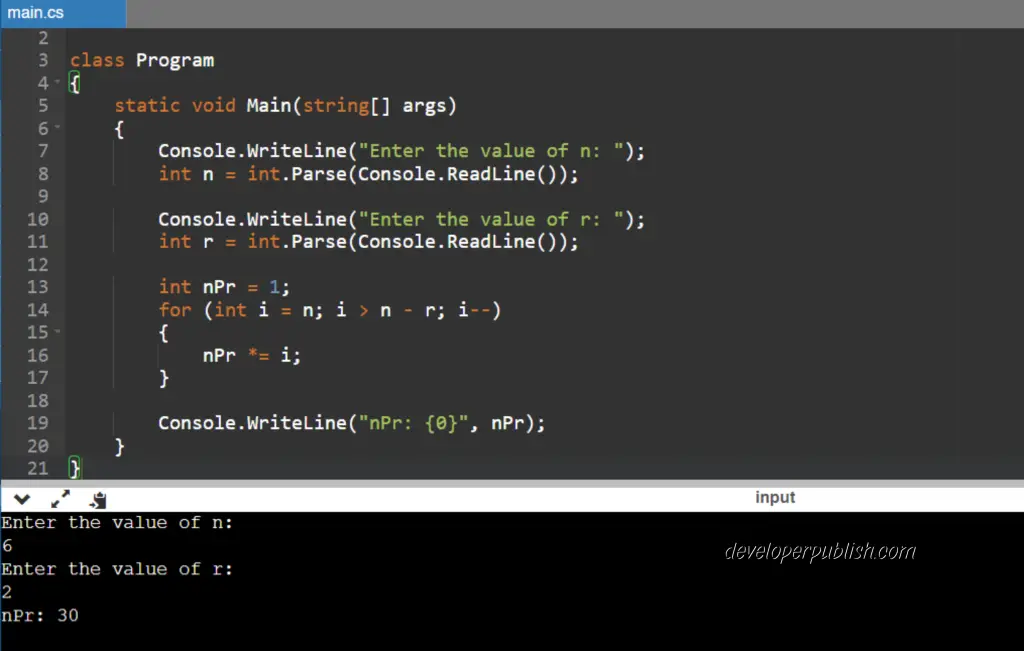