This C# program calculates and prints the edge values of a power function.
Problem statement
Given a base and an exponent, this program calculates the edge values\n” + “of the power function for a range of inputs and prints the results.
C# Program to Print the Edge Values in Power Function
using System; class Program { static void Main() { // Introduction Console.WriteLine("Power Function Edge Values Calculator"); Console.WriteLine("------------------------------------\n"); // Problem Statement Console.WriteLine("Given a base and an exponent, this program calculates the edge values\n" + "of the power function for a range of inputs and prints the results.\n"); // Input Console.Write("Enter the base: "); double baseValue = double.Parse(Console.ReadLine()); Console.Write("Enter the exponent: "); int exponent = int.Parse(Console.ReadLine()); Console.Write("Enter the starting value for the range: "); double startValue = double.Parse(Console.ReadLine()); Console.Write("Enter the ending value for the range: "); double endValue = double.Parse(Console.ReadLine()); // Program Console.WriteLine("\nResults:"); for (double x = startValue; x <= endValue; x++) { double result = Math.Pow(baseValue, x); Console.WriteLine($"Base^{x} = {result}"); } // How it works Console.WriteLine("\nHow It Works:"); Console.WriteLine("1. You enter the base and exponent for the power function."); Console.WriteLine("2. You specify a range of input values."); Console.WriteLine("3. The program calculates and prints the result of the power function for each value in the specified range."); } }
How it works
- You enter the base and exponent for the power function.
- You specify a range of input values.
- The program calculates and prints the result of the power function for each value in the specified range.
Input / output
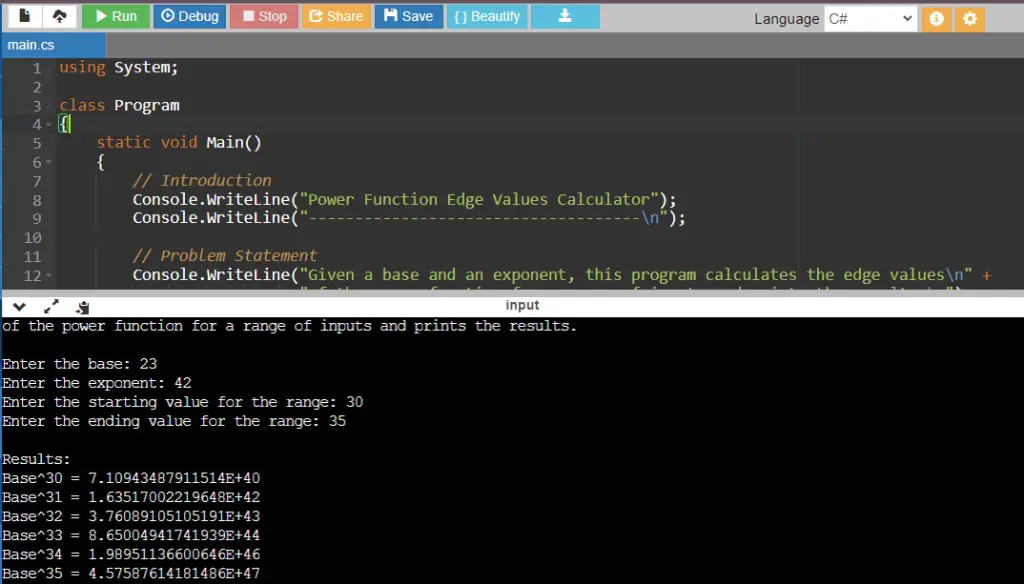