In statistics, the mean is the average of a set of numbers. In this task, we will write a C# program to find the mean of a set of numbers.
Problem Statement
Write a C# program to find the mean of a set of numbers. The program should take input from the user in the form of a set of numbers. The program should display the mean of the numbers as output.
C# Program to Find the Mean
using System; class Program { static void Main(string[] args) { Console.WriteLine("Enter the numbers separated by spaces: "); string input = Console.ReadLine(); string[] numbers = input.Split(' '); double sum = 0; foreach (string number in numbers) { sum += double.Parse(number); } double mean = sum / numbers.Length; Console.WriteLine("Mean: {0}", mean); } }
Input / Output
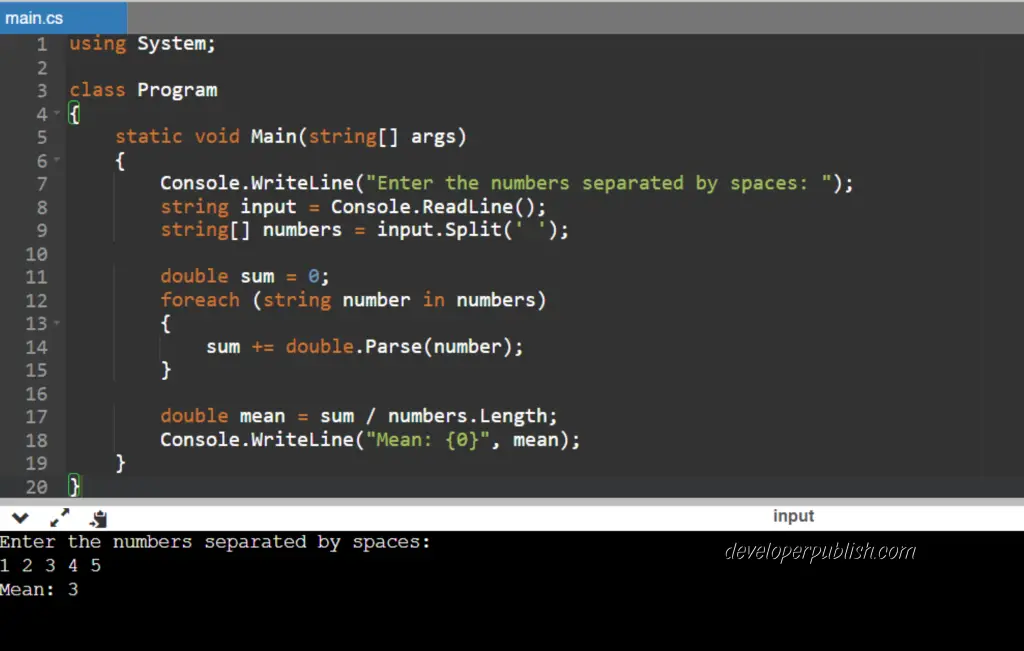