This C# console program simulates an ATM (Automated Teller Machine) interface for basic transactions. It allows users to perform the following operations.
Problem Statement
You are tasked with developing a simple ATM (Automated Teller Machine) transaction program in C#. The program should allow users to interact with their bank accounts by performing the following operations:
- Check Balance: Users should be able to check their account balance.
- Deposit: Users should be able to deposit money into their account.
- Withdraw: Users should be able to withdraw money from their account, provided they have sufficient funds.
- Exit: Users should have the option to exit the program.
C# Program to Display the ATM Transaction
using System; class Program { static double balance = 1000.00; // Initial balance static void Main(string[] args) { while (true) { Console.WriteLine("ATM Menu:"); Console.WriteLine("1. Check Balance"); Console.WriteLine("2. Deposit"); Console.WriteLine("3. Withdraw"); Console.WriteLine("4. Exit"); Console.Write("Enter your choice: "); if (int.TryParse(Console.ReadLine(), out int choice)) { switch (choice) { case 1: CheckBalance(); break; case 2: Deposit(); break; case 3: Withdraw(); break; case 4: Console.WriteLine("Thank you for using our ATM. Goodbye!"); return; default: Console.WriteLine("Invalid choice. Please try again."); break; } } else { Console.WriteLine("Invalid input. Please enter a valid number."); } } } static void CheckBalance() { Console.WriteLine($"Your balance: ${balance:F2}"); } static void Deposit() { Console.Write("Enter the deposit amount: $"); if (double.TryParse(Console.ReadLine(), out double amount) && amount > 0) { balance += amount; Console.WriteLine($"Deposit successful. Your new balance: ${balance:F2}"); } else { Console.WriteLine("Invalid amount. Please enter a valid positive number."); } } static void Withdraw() { Console.Write("Enter the withdrawal amount: $"); if (double.TryParse(Console.ReadLine(), out double amount) && amount > 0) { if (balance >= amount) { balance -= amount; Console.WriteLine($"Withdrawal successful. Your new balance: ${balance:F2}"); } else { Console.WriteLine("Insufficient funds. Your balance is not enough for this withdrawal."); } } else { Console.WriteLine("Invalid amount. Please enter a valid positive number."); } } }
How it Works
- Initialization: The program begins by initializing a
balance
variable to an initial value of $1000. This represents the starting balance in the user’s account. - Welcome Message: The program displays a welcome message and an introduction explaining the purpose of the program and its available features.
- Menu Display and Input: The program enters a
while
loop that presents the user with an ATM menu containing four options:- Check Balance (Option 1): Allows the user to check their account balance.
- Deposit (Option 2): Allows the user to deposit money into their account.
- Withdraw (Option 3): Allows the user to withdraw money from their account.
- Exit (Option 4): Allows the user to exit the program.
- User Input: The program prompts the user to enter their choice (a number between 1 and 4). It then reads and validates the user’s input.
- Switch Statement: The program uses a
switch
statement to perform different actions based on the user’s choice:- Option 1 (Check Balance): It calls the
CheckBalance
function to display the current account balance. - Option 2 (Deposit): It calls the
Deposit
function to allow the user to deposit money into their account. - Option 3 (Withdraw): It calls the
Withdraw
function to allow the user to withdraw money from their account. - Option 4 (Exit): It exits the program with a thank-you message if the user chooses to exit.
- Option 1 (Check Balance): It calls the
- Check Balance (Option 1): The
CheckBalance
function simply displays the current account balance. - Deposit (Option 2): The
Deposit
function prompts the user to enter the amount they want to deposit. It validates the input to ensure it’s a positive number and then adds the deposited amount to the account balance, displaying the updated balance. - Withdraw (Option 3): The
Withdraw
function prompts the user to enter the amount they want to withdraw. It validates the input, checks if the balance is sufficient, and subtracts the withdrawal amount from the account balance if possible. It then displays the updated balance or an error message if the withdrawal is not possible due to insufficient funds. - Exit (Option 4): If the user chooses to exit the program, it displays a thank-you message and terminates the program.
- Loop Continuation: After each operation (checking balance, depositing, withdrawing, or exiting), the program returns to the ATM menu, allowing the user to perform additional transactions or exit the program.
Input/ Output
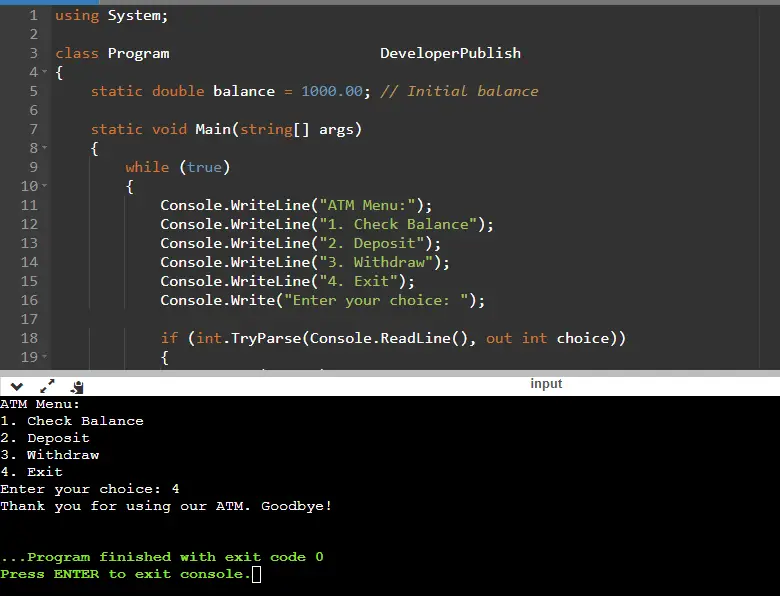