This program adds two binary numbers in the C programming language.
Problem Statement
Given two binary numbers as input, write a C program to add them and print the result.
Solution
To solve this problem, we will first convert the binary numbers to decimal numbers using the following algorithm:
- Initialize a variable
result
to 0. - Iterate over each digit of the binary number from right to left.
- For each digit, if it is 1, add 2^(index) to
result
, whereindex
is the position of the digit starting from 0. - Return the final value of
result
.
Once we have converted both binary numbers to decimal numbers, we can simply add them using the +
operator and convert the result back to a binary number. Here’s the code:
#include <stdio.h> int binaryToDecimal(long long binary); long long decimalToBinary(int decimal); int main() { long long binary1, binary2; int decimal1, decimal2, sum; printf("Enter the first binary number: "); scanf("%lld", &binary1); printf("Enter the second binary number: "); scanf("%lld", &binary2); decimal1 = binaryToDecimal(binary1); decimal2 = binaryToDecimal(binary2); sum = decimal1 + decimal2; printf("Sum of %lld and %lld is %lld in binary.\n", binary1, binary2, decimalToBinary(sum)); return 0; } int binaryToDecimal(long long binary) { int decimal = 0, index = 0; while (binary != 0) { int digit = binary % 10; decimal += digit * (1 << index); binary /= 10; index++; } return decimal; } long long decimalToBinary(int decimal) { long long binary = 0, place = 1; while (decimal != 0) { binary += (decimal % 2) * place; decimal /= 2; place *= 10; } return binary; }
Let’s go through the code step-by-step:
- We include the standard input/output header file
stdio.h
. - We declare two functions called
binaryToDecimal()
anddecimalToBinary()
. ThebinaryToDecimal()
function takes a long long integer argumentbinary
and returns its decimal equivalent. ThedecimalToBinary()
function takes an integer argumentdecimal
and returns its binary equivalent as a long long integer. - In the
main()
function, we declare four variables:binary1
andbinary2
are the two binary numbers entered by the user,decimal1
anddecimal2
are their decimal equivalents, andsum
is their sum in decimal form. - We prompt the user to enter the two binary numbers using the
printf()
andscanf()
functions. - We convert both binary numbers to decimal form using the
binaryToDecimal()
function. - We add the decimal equivalents of the binary numbers using the
+
operator to get their sum in decimal form. - We convert the decimal sum back to a binary number using the
decimalToBinary()
function and print it using theprintf()
function.
The binaryToDecimal()
function takes a binary number as input and converts it to decimal form using the algorithm described above. It iterates over each digit of the binary number from right to left using a while loop. For each digit, it multiplies 2^(index) by the value of the digit (0 or 1) and adds the result to
the decimal
variable. It also increments the index
variable for each digit. Finally, it returns the decimal equivalent of the binary number.
The decimalToBinary()
function takes a decimal number as input and converts it to binary form using the algorithm described above. It iterates over each bit of the binary number from right to left using a while loop. For each bit, it computes the remainder of the division by 2 (0 or 1) and multiplies it by a place value (place
) that starts at 1 and doubles with each iteration. It adds the result to the binary
variable and divides the decimal number by 2, discarding the remainder. Finally, it returns the binary equivalent of the decimal number.
Output
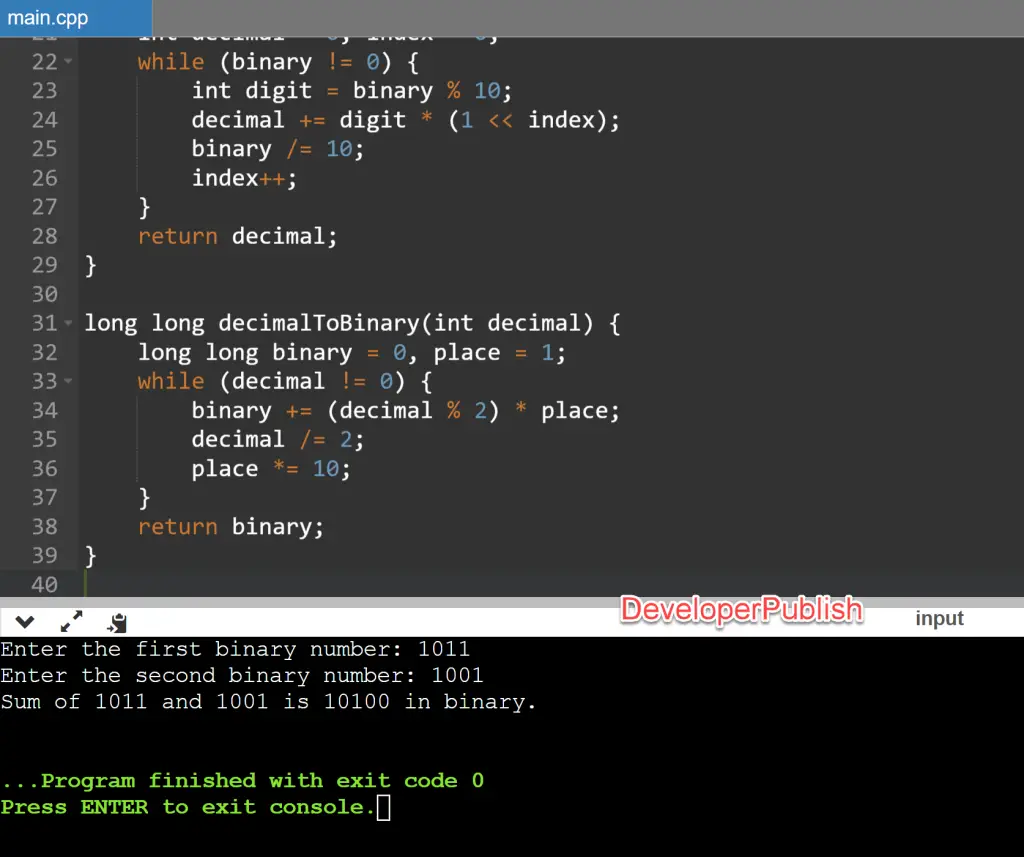
Explanation
Let’s say the user enters the binary numbers 1101 and 10011. The main()
function prompts the user to enter the two binary numbers using the printf()
and scanf()
functions. Then it calls the binaryToDecimal()
function to convert the binary numbers to decimal form and adds them using the +
operator to get their sum in decimal form.
The binaryToDecimal()
function converts the first binary number (1101) to its decimal equivalent (13) using the following algorithm:
- The
decimal
variable is initialized to 0 and theindex
variable is initialized to 0. - The while loop iterates as long as the binary number is not 0.
- The
digit
variable is set to the remainder of the binary number divided by 10 (which is 1). - The decimal equivalent of the digit is computed by multiplying it by 2^(index) (which is 1, since index is 0).
- The result is added to the
decimal
variable (which becomes 1). - The binary number is divided by 10, discarding the last digit (which becomes 110).
- The
index
variable is incremented by 1 (which becomes 1). - Steps 3-7 are repeated for each digit of the binary number.
- The
decimal
variable is returned (which is 13).
The binaryToDecimal()
function then converts the second binary number (10011) to its decimal equivalent (19) using the same algorithm.
The main()
function adds the decimal equivalents of the two binary numbers (13 + 19 = 32) and calls the decimalToBinary()
function to convert the decimal sum back to a binary number.
The decimalToBinary()
function converts the decimal number 32 to its binary equivalent (100000) using the following algorithm:
- The
binary
variable is initialized to 0 and theplace
variable is initialized to 1. - The while loop iterates as long as the decimal number is not 0.
- The remainder of the decimal number divided by 2 (which is 0) is computed and multiplied by the
place
variable (which is 1). - The result is added to the
binary
variable (which becomes 0). - The decimal number is divided by 2, discarding the remainder (which becomes 16).
- The
place
variable is doubled (which becomes 2). - Steps 3-6 are repeated for each bit of the binary number.
- The
binary
variable is returned (which is 100000).
The main()
function then prints the binary sum using the printf()
function (which is 100000 in this case).
Conclusion
In this program, we have seen how to add two binary numbers in the C programming language. We first converted the binary numbers to decimal form , then added them using the +
operator, and finally converted the decimal sum back to binary form. We used two separate functions to convert binary to decimal and decimal to binary, each implementing a different algorithm. The program demonstrates the use of loops, variables, and functions in C, as well as the conversion between different number systems.