Everwondered how you can use a ternary operator with-in a string interpolation in C#?. This simple tip shows you how you can do it.
Assume that you have a variable isArchitect and you would like to set a text Yes when the value is set with-in the interpolated string.
One of the simplest option is to first use the ternary operator and set the string as shown below.
var architectResult = isArchitect ? " Yes" : "No"; var result = $"{fieldName}{architectResult}";
How to use Ternary Operator in Interpolated String in C# ?
If you need them in the single line with-in the string interpolation, you will need to wrap the condition in parenthesis as shown below.
var result = $"Architect : {(isArchitect ? "yes" : "no")}";
Here’s the complete code snippet demonstrating the usage of ternary operator with-in the interpolation string.
public class Hello { public static void Main() { var isArchitect = true; var result = $"Architect : {(isArchitect ? "yes" : "no")}"; System.Console.WriteLine(result); } }
Output
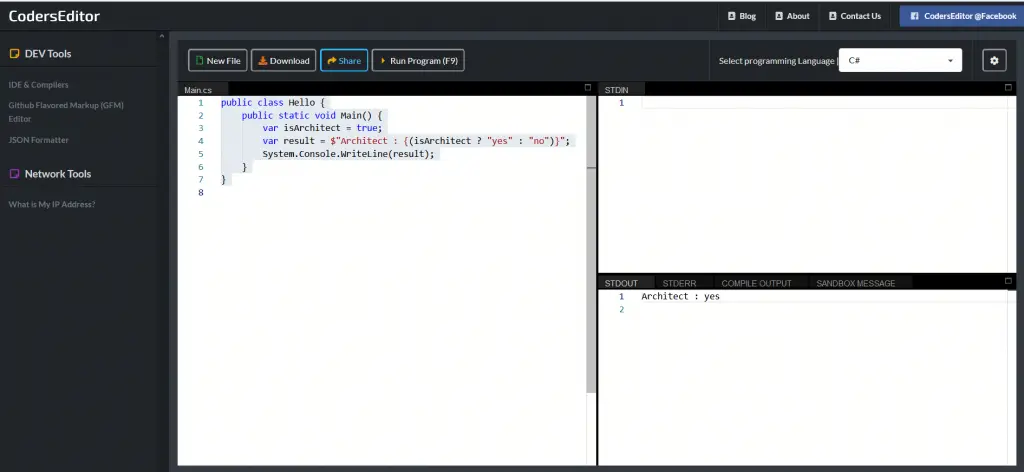