This blog post will provide a simple tip demonstrating how you can implement a truncate function in C# so that value in a string gets truncated based on the given length.
How to Truncate a string in C#?
Out of the box , C# does not have any extension method called Truncate that works for this scenario. However, you can build a simple extension method in C# to truncate a string.
Here’s the Extension method that uses the Substring to fetch and truncate the results.
public static class StringExtension { public static string Truncate(this string input, int strLength) { if (string.IsNullOrEmpty(input)) return input; return input.Length <= strLength ? input : input.Substring(0, strLength); } }
Complete Code Snippet is below.
public class Hello { public static void Main() { string input = "Welcome to DeveloperPublish.com"; string truncatedString = input.Truncate(10); System.Console.WriteLine(truncatedString); } } public static class StringExtension { public static string Truncate(this string input, int strLength) { if (string.IsNullOrEmpty(input)) return input; return input.Length <= strLength ? input : input.Substring(0, strLength); } }
Output
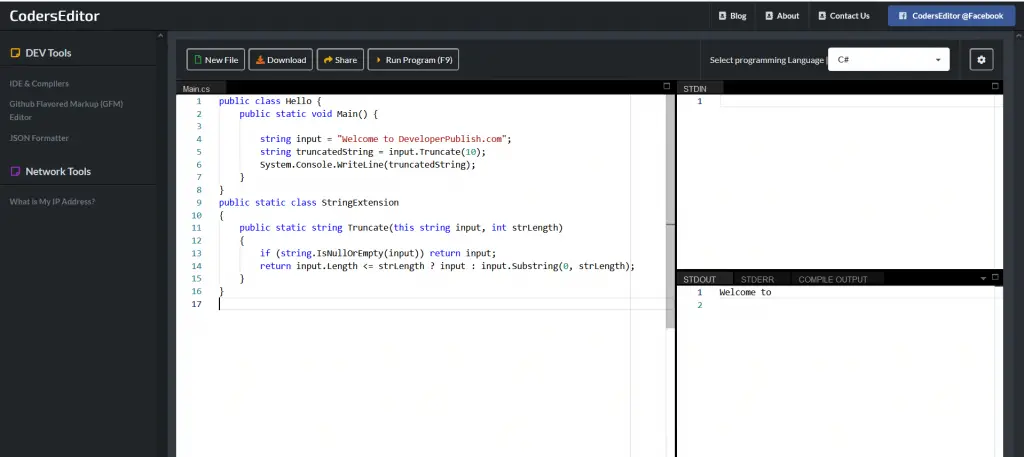