This Python program finds all Pythagorean triplets within a given range of positive integers. Pythagorean triplets are sets of three numbers that satisfy the Pythagorean theorem.
Problem statement
Write a Python program that takes a range of positive integers as input and finds all the Pythagorean triplets within that range. The program should output the list of triplets found.
Python Program to Find All Pythagorean Triplets in the Range
def find_pythagorean_triplets(range_limit): triplets = [] for a in range(1, range_limit+1): for b in range(a+1, range_limit+1): c = (a**2 + b**2) ** 0.5 if c.is_integer() and c <= range_limit: triplets.append((a, b, int(c))) return triplets # Take user input for the range limit range_limit = int(input("Enter the range limit: ")) # Find Pythagorean triplets within the given range triplets = find_pythagorean_triplets(range_limit) # Output the triplets print("Pythagorean triplets within the range {}:".format(range_limit)) for triplet in triplets: print(triplet)
Input/output
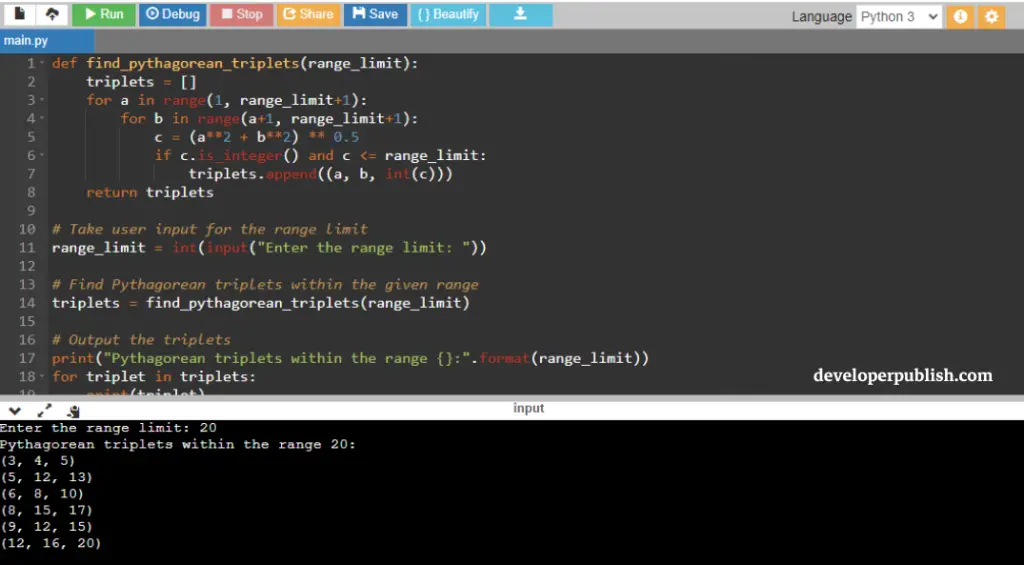