Polynomial equations are fundamental mathematical expressions used in a wide range of applications. This Python program computes the value of a polynomial equation, allowing users to input the coefficients and a specific value for the variable.
Problem statement
Given a polynomial equation of any degree, expressed as y = a * x^3 + b * x^2 + c * x + d
, the program aims to calculate the value of y
by taking user inputs for the coefficients a
, b
, c
, d
, and the value of x
.
Python Program to Compute a Polynomial Equation
# Function to compute the polynomial equation def compute_polynomial(a, b, c, d, x): return a * x**3 + b * x**2 + c * x + d # Taking input from the user a = float(input("Enter the coefficient of x^3: ")) b = float(input("Enter the coefficient of x^2: ")) c = float(input("Enter the coefficient of x: ")) d = float(input("Enter the constant term: ")) x = float(input("Enter the value of x: ")) # Computing the polynomial equation result = compute_polynomial(a, b, c, d, x) # Displaying the result print("The value of the polynomial equation is:", result)
Input\Output
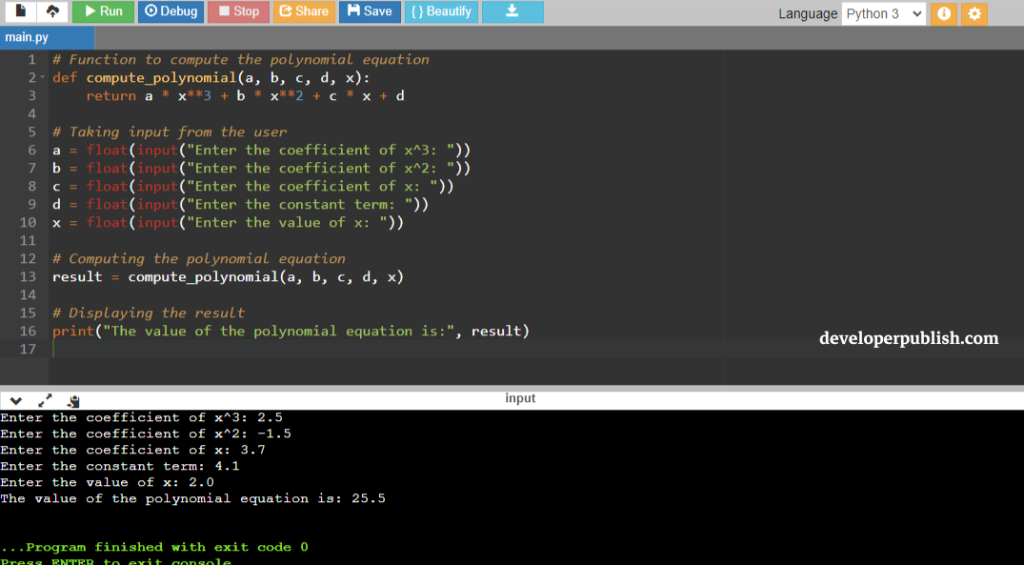