Here’s a Python program to calculate simple interest with an introduction:
Simple interest is a formula used to calculate the interest earned or paid on a principal amount over a specified period of time. The formula for calculating simple interest is:
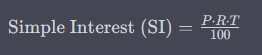
Where:
- SISI is the simple interest.
- PP is the principal amount.
- RR is the rate of interest per annum.
- TT is the time (in years) for which interest is calculated.
Problem statement
Write a Python program that calculates the simple interest based on the principal amount, interest rate, and time period provided by the user. The program should take the input values, perform the necessary calculations, and display the simple interest as the output.
Python Program to Find Simple Interest
# Python Program to Find Simple Interest # Function to calculate simple interest def calculate_simple_interest(principal, rate, time): interest = (principal * rate * time) / 100 return interest # Get input from the user principal = float(input("Enter the principal amount: ")) rate = float(input("Enter the interest rate: ")) time = float(input("Enter the time period (in years): ")) # Calculate simple interest simple_interest = calculate_simple_interest(principal, rate, time) # Display the result print("Simple Interest:", simple_interest)
Input/Output
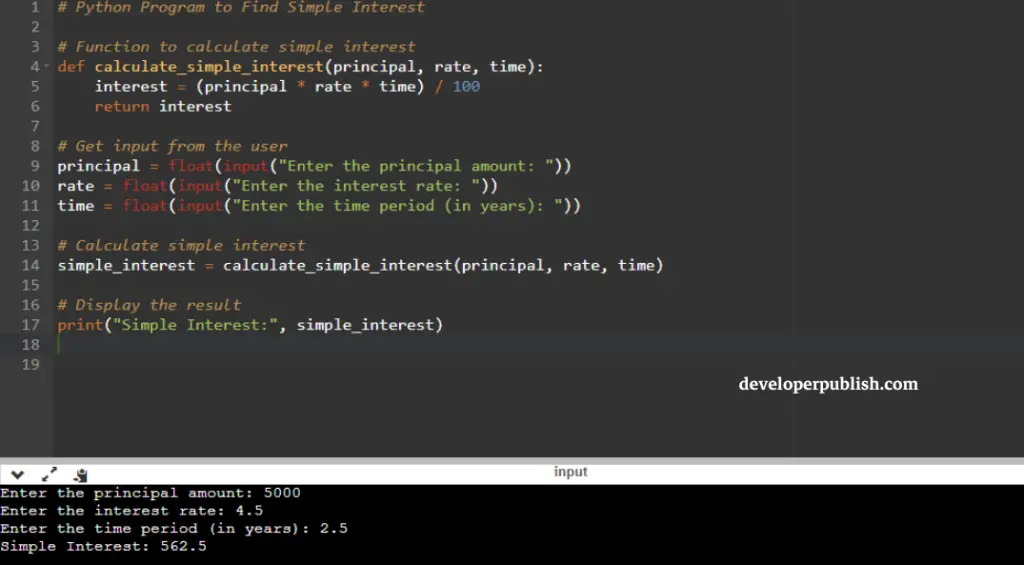