Sorting a list of tuples in increasing order by the last element in each tuple is a common task in programming. Tuples are ordered collections of elements, and sometimes you might want to sort a list of tuples based on a specific element within each tuple.
Problem Statement
You are given a list of tuples, where each tuple contains a sequence of elements. Your task is to implement a function in Python that sorts this list of tuples in increasing order based on the last element of each tuple.
Python Program to Sort a List of Tuples in Increasing Order by the Last Element in Each Tuple
def sort_tuples_by_last_element(lst): return sorted(lst, key=lambda x: x[-1]) # Example list of tuples tuple_list = [(1, 4), (3, 2), (8, 9), (5, 7)] sorted_list = sort_tuples_by_last_element(tuple_list) print(sorted_list)
How it Works
- Defining the Function: We start by defining the
sort_tuples_by_last_element(lst)
function. This function takes a list of tupleslst
as an argument. - Using the
sorted()
Function: Inside the function, we use thesorted()
function to perform the sorting. Thesorted()
function takes two important parameters:iterable
: This is the list of tuples we want to sort.key
: This is a function that takes an element from theiterable
and returns a value based on which the sorting is done.
- Lambda Function for Sorting: In the
key
parameter of thesorted()
function, we use a lambda function to specify that we want to sort based on the last element of each tuple. The lambda functionlambda x: x[-1]
takes a tuplex
and returns its last element, which is used for sorting. - Result: The
sorted()
function returns a new list containing the sorted tuples. This list is assigned to thesorted_list
variable. - Returning the Result: Finally, the
sort_tuples_by_last_element()
function returns thesorted_list
.
Input/ Output
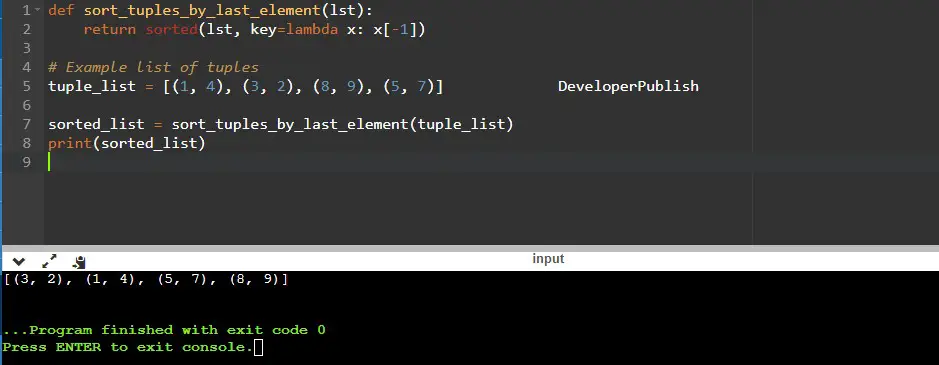