In this program, we will write a Python code to find the smallest divisor of a given integer. The divisor is a number that divides another number without leaving a remainder. We will use a brute-force approach to find the smallest divisor by iterating through all numbers starting from 2 and checking if they evenly divide the given integer.
Problem Statement
Write a Python program to find the smallest divisor of a given integer.
Python Program to Find the Smallest Divisor of an Integer
def smallest_divisor(n): """ Function to find the smallest divisor of a given integer. """ # Check if the number is less than 2 if n < 2: return None # Iterate through all numbers starting from 2 for i in range(2, int(n**0.5) + 1): # Check if i is a divisor of n if n % i == 0: return i # If no divisor is found, the number itself is the smallest divisor return n # Get user input num = int(input("Enter an integer: ")) # Find the smallest divisor result = smallest_divisor(num) # Print the result if result is None: print("No smallest divisor exists for the given number.") else: print(f"The smallest divisor of {num} is {result}.")
How it works
- The function
smallest_divisor
takes an integern
as input and returns the smallest divisor of that number. - It first checks if the number is less than 2. If it is, the function returns
None
as there is no divisor less than 2 for any number. - It then iterates through all numbers starting from 2 up to the square root of
n
(rounded up to the nearest integer). - For each number
i
, it checks ifi
is a divisor ofn
by using the modulo operator (%
). Ifn
is divisible byi
, it meansi
is the smallest divisor ofn
, and the function returnsi
. - If no divisor is found in the previous step, it means that the number itself is the smallest divisor, and the function returns
n
. - In the main program, the user is prompted to enter an integer.
- The
smallest_divisor
function is called with the user input as the argument, and the result is stored in theresult
variable. - Finally, the program checks if the
result
isNone
(indicating no smallest divisor) and prints the appropriate message. Otherwise, it prints the smallest divisor found.
Input / Output
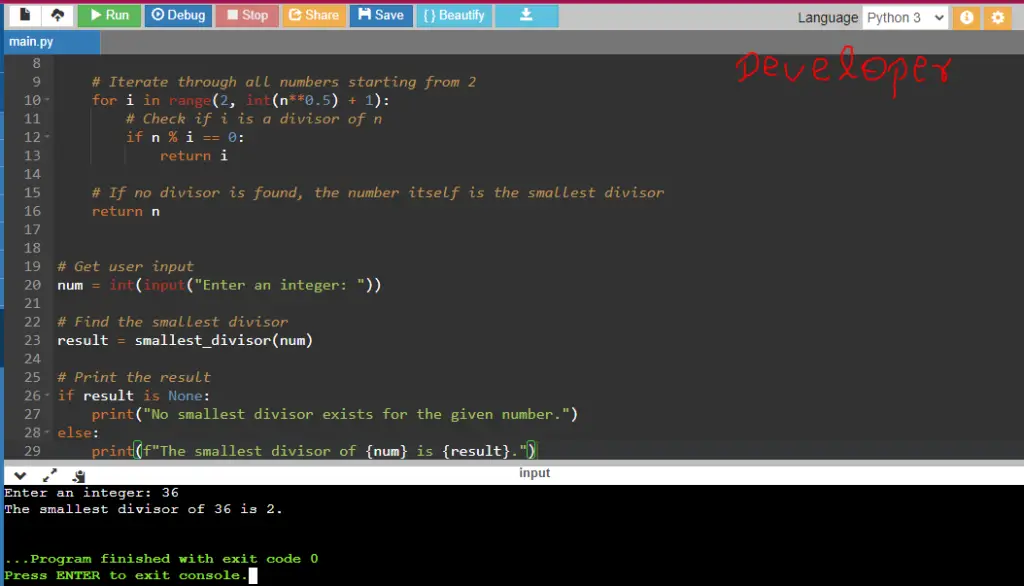