This Python program calculates the sum of the first N natural numbers. It defines a function called calculat_sum
that takes an integer n
as input and uses a for
loop to iterate from 1 to n
, adding each number to the sum. The program then prompts the user to enter a positive integer n
, calls the calculate_sum
function, and prints the result. It’s important to note that the program assumes the user will provide a positive integer as input.
Problem Statement
Write a Python program that calculates the sum of the first N natural numbers.
Inputs:
- An integer N representing the count of natural numbers to be considered.
Output:
- The sum of the first N natural numbers.
Python Program to Find Sum of First N Natural Numbers
def calculate_sum(n): sum = 0 for i in range(1, n + 1): sum += i return sum # Example usage n = int(input("Enter a positive integer: ")) result = calculate_sum(n) print(f"The sum of the first {n} natural numbers is {result}.")
How it Works
The Python program to calculate the sum of the first N natural numbers works as follows:
- It defines a function called
calculate_sum
that takes an integern
as input. - Inside the
calculate_sum
function, it initializes a variablesum
to 0. This variable will store the sum of the natural numbers. - It uses a
for
loop with therange()
function to iterate from 1 ton+1
. The loop variablei
represents each natural number. - Within each iteration of the loop, it adds the current value of
i
to thesum
variable. - After the loop completes, it returns the final value of
sum
. - The program prompts the user to enter a positive integer
N
using theinput()
function. - It converts the user input to an integer using the
int()
function and assigns it to the variablen
. - It calls the
calculate_sum
function with the value ofn
as an argument and assigns the result to the variableresult
. - Finally, it prints the sum of the first N natural numbers using a formatted string, combining the value of
n
andresult
.
Input/ Output
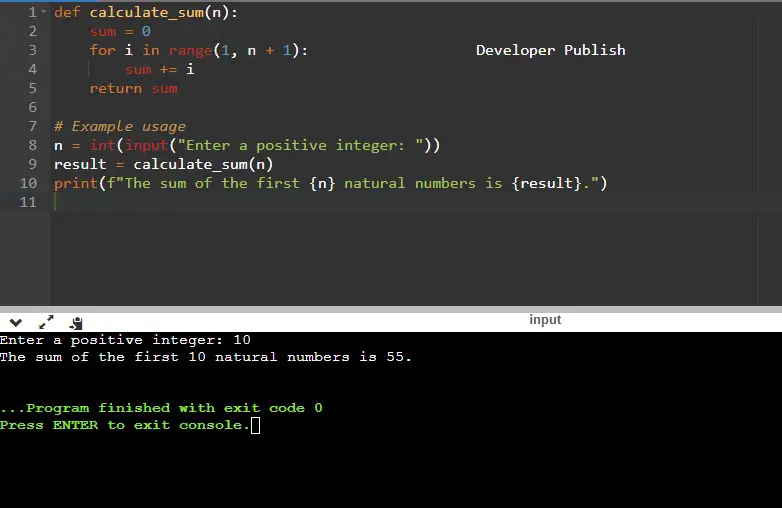