If you are looking at a way to get the name of the current method in C#, here’s a quick tip in C# on how to do it with few options.
How to Get Name of the Current Method Using in C# ?
Option 1 : Using Reflection
One of the easiest way to do it is using reflection. The Reflection name space provides the MethodBase class which exposes the GetCurrentMethod to get the method information.
System.Reflection.MethodBase.GetCurrentMethod().Name;
Here’s a sample code snippet in C# demonstrating how to get the current method name using reflection.
using System; namespace AbundantCode { internal class Program { private static void Main(string[] args) { var MethodName = System.Reflection.MethodBase.GetCurrentMethod().Name; Console.WriteLine(MethodName); Console.ReadLine(); } } }
Note : This would work for non-sync methods.
Option 2 : Using the StackTrace Class.
The Other option to get the name of the method is using the StackTrace method and getting the stack frame to fetch the method information as shown in the below code snippet.
using System; using System.Diagnostics; namespace AbundantCode { internal class Program { private static void Main(string[] args) { var sTrace = new StackTrace(); var Frame = sTrace.GetFrame(0); var currentMethodName = Frame.GetMethod().Name; Console.WriteLine(currentMethodName); Console.ReadLine(); } } }
Output
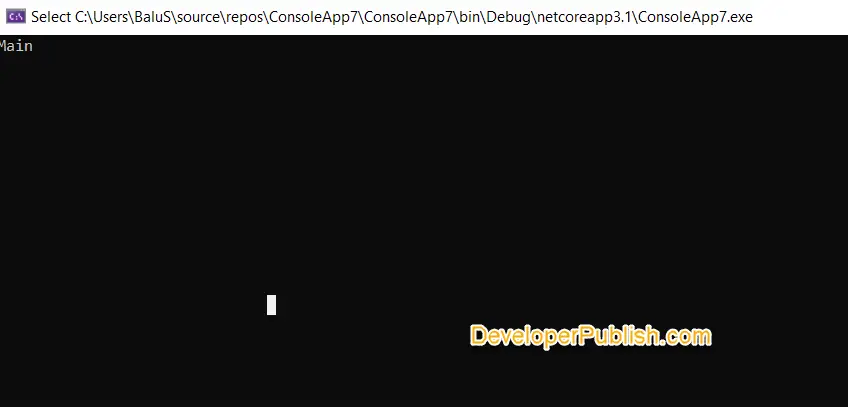