In the realm of student management systems, the assignment of unique register numbers is a fundamental task. Automation plays a crucial role in ensuring efficiency and accuracy in this process. In this C# program, we delve into the world of object-oriented programming to accomplish precisely that.
Problem statement
Design a C# program that automates the process of generating unique register numbers for a group of 100 students. This program should utilize a static constructor to ensure efficiency and accuracy in the registration process.
C# Program to Generate Register Number Automatically for 100 Students using Static Constructor
using System; class Student { private static int counter = 1000; private string registerNumber; private string name; // Static constructor to initialize the counter static Student() { counter = 1000; // Start with 1000 } public Student(string name) { this.name = name; this.registerNumber = "R" + counter.ToString(); counter++; } public void DisplayDetails() { Console.WriteLine("Name: " + name); Console.WriteLine("Register Number: " + registerNumber); } } class Program { static void Main() { Student[] students = new Student[100]; for (int i = 0; i < 100; i++) { students[i] = new Student("Student " + (i + 1)); } // Display details for all students foreach (var student in students) { student.DisplayDetails(); Console.WriteLine(); } } }
How it works
Sure, I’ll explain how the C# program works step by step:
- Student Class:
- The program starts by defining a
Student
class. This class represents a student and has two private fields:registerNumber
(to store the generated register number) andname
(to store the name of the student). - Additionally, it has a static field called
counter
which will be used to generate unique register numbers. - The static constructor
static Student()
initializes thecounter
to 1000. This is only executed once, the first time any member of the class is accessed. - There is a constructor
public Student(string name)
which takes the name of the student as an argument. It assigns a register number in the format “RXXXX” where XXXX is the current value of the counter. DisplayDetails()
is a method that displays the name and register number of the student.
- The program starts by defining a
- Main Method:
- In the
Main
method, an array ofStudent
objects (students
) is created to hold information for 100 students. - A loop iterates 100 times to create 100 student objects. For each student, a new
Student
object is created, and a register number is automatically generated. - After creating the students, another loop is used to display the details of each student.
- In the
- Execution:
- When the program is executed, the static constructor is called automatically before any other members of the class are accessed.
- As students are created, their register numbers are assigned automatically, starting from R1000 and incrementing for each new student.
- Finally, the details of all 100 students are displayed, showing their names and respective register numbers.
- Output:
- The program outputs the details of all 100 students, demonstrating the successful automatic generation of register numbers.
By utilizing a static constructor, the program ensures that the counter is initialized only once, and every new student object receives a unique register number. This approach encapsulates the register number generation logic within the Student
class, promoting clean and efficient code.
Input/Output
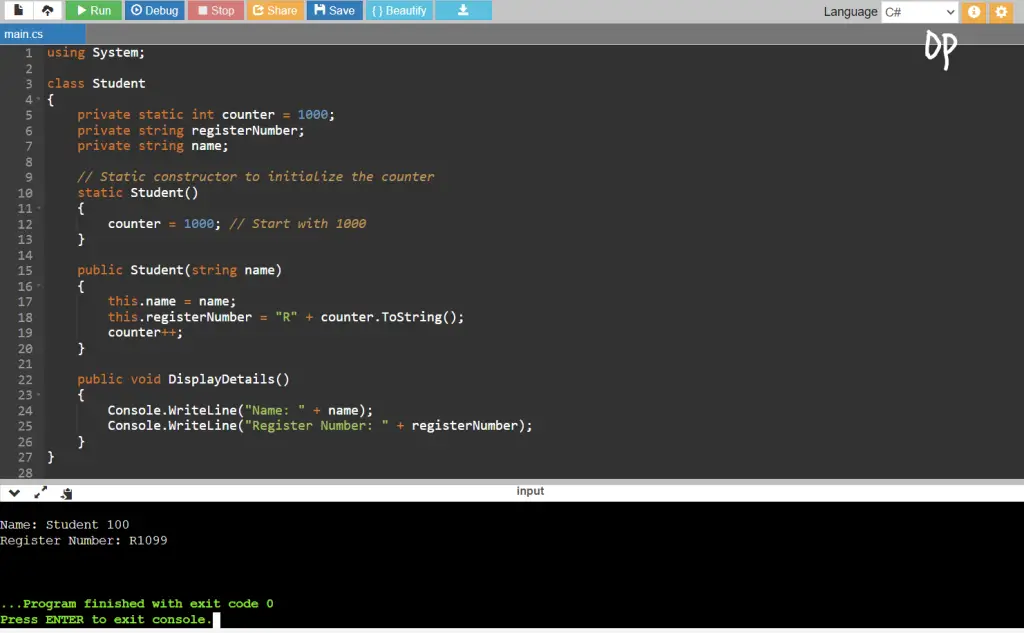